Table of Contents
In the ever-evolving world of WordPress development, the importance of using JavaScript and the WordPress REST API cannot be overstated. As the digital landscape becomes increasingly interactive and user-centric, these two technologies have emerged as vital tools for creating dynamic, responsive, and engaging web experiences.
JavaScript, a high-level, interpreted programming language, is a key player in the development of interactive web elements. It’s used to enhance user interfaces and create rich, web-based applications. On the other hand, the WordPress REST API is a feature of WordPress that provides a standardized way for developers to interact with the site’s content and features. It allows developers to send and receive data as JSON (JavaScript Object Notation) objects, thereby enabling the creation of powerful interfaces for managing and publishing site content.
The WordPress REST API is the foundation of the WordPress Block Editor and can enable your theme, plugin, or custom application to present new, powerful interfaces for managing and publishing your site content. It is a developer-oriented feature of WordPress that provides data access to the content of your site and implements the same authentication restrictions. This means that content that is public on your site is generally publicly accessible via the REST API, while private content, password-protected content, internal users, custom post types, and metadata are only available with authentication or if you specifically set it to be so.
In essence, the combination of JavaScript and the WordPress REST API has revolutionized the way developers approach WordPress development, opening up new possibilities for creating more interactive and user-friendly websites. In this article, we will delve deeper into these technologies and explore the best practices for using them effectively.
Sources:
REST API Handbook – WordPress Developer Resources
Understanding the WordPress REST API
The WordPress REST API is a transformative feature that has significantly changed the way developers interact with WordPress. It has opened up new possibilities for creating dynamic, interactive websites and applications, and has made WordPress more than just a content management system.
The REST API allows developers to interact with WordPress from outside the WordPress installation itself. This means that a third-party website or a mobile app, for example, can access your WordPress database, fetch data from it, and add data to it. This is achieved by sending and receiving data as JSON (JavaScript Object Notation) objects.
The principles of REST (Representational State Transfer) are integral to understanding how the WordPress REST API operates. REST provides standards that web systems can use to interface with each other. Without REST, two systems wouldn’t be able to understand each other and so send data back and forth.
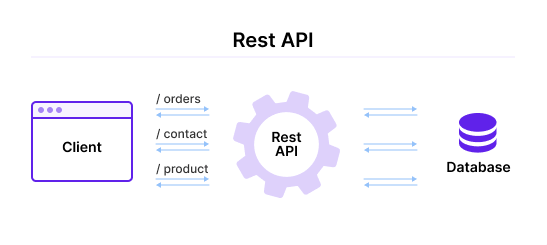
For an application to be RESTful, it must conform to five principles:
- Uniform interface: The URLs used to access resources in the system have to be uniform, consistent, and accessible via a common approach such as GET.
- Client-server: Client applications and server applications must be separate, so they can be developed independently of each other. If the server-side technology (i.e., WordPress) changes, the client-side application (an app, for example) must still be able to access it via the same simple method.
- Stateless: The server doesn’t change state when a new request is made using the API. It doesn’t store the requests that have been made.
- Cacheable: All resources must be cacheable, to improve speed and conformance to web standards. Caching can be implemented on the server or client-side.
- Layered system: A RESTful system lets you use multiple layers to access it, storing data in intermediate servers if it needs to. The server can’t tell if the final client is directly connected to it.
These principles ensure that the WordPress REST API is scalable, flexible, and can be used with any programming language or platform, making it a powerful tool for developers.
In the next sections, we will delve deeper into how to use JavaScript with the WordPress REST API effectively, and provide some practical examples and code snippets to illustrate these concepts.
Sources:
The Complete Guide to WordPress REST API Basics
WordPress REST API For the Enterprise | The Agile Content Platform
Designing Your First REST API – Part 1 | Everything I Know
Using JavaScript with the WordPress REST API
JavaScript has become a fundamental part of modern web development, and its use with the WordPress REST API opens up a world of possibilities for creating interactive websites and applications.
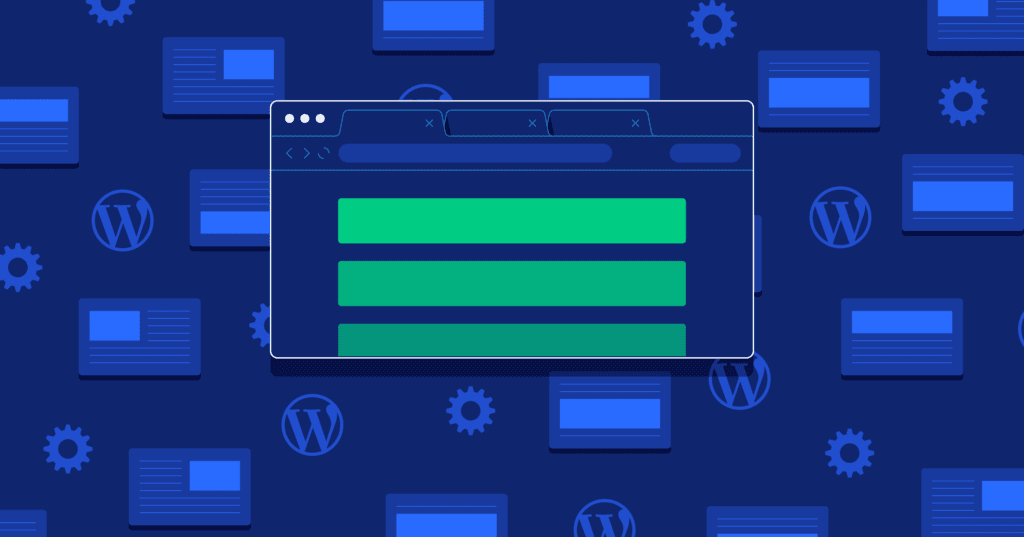
Why Use JavaScript with the REST API?
JavaScript is a powerful language that allows developers to create dynamic and interactive web applications. When used with the WordPress REST API, JavaScript can help create a more engaging and responsive user experience.
The WordPress REST API provides an interface for applications to interact with your WordPress site by sending and receiving data as JSON (JavaScript Object Notation) objects. This means that you can use JavaScript to interact with your WordPress site’s data in a structured and predictable way, without needing to reload the page.
How JavaScript Creates Interactive Websites and Apps Using the REST API
JavaScript can interact with the REST API to create, read, update, and delete data on your WordPress site. This can be done through AJAX (Asynchronous JavaScript and XML) calls, which allow data to be exchanged with a server and update parts of a web page without reloading the whole page.
For example, you could use JavaScript and the REST API to create a live search feature on your WordPress site. As a user types into the search bar, JavaScript can make requests to the REST API to fetch relevant posts and display them on the page, all without the need for a page refresh.
Examples of Using JavaScript and the REST API in WordPress
There are many ways you can use JavaScript and the REST API together in WordPress. Here are a few examples:
- Creating a Single Page Application (SPA): SPAs are web applications that load a single HTML page and dynamically update that page as the user interacts with the app. With JavaScript and the REST API, you can create a SPA within your WordPress site, where each “page” is loaded dynamically without a page refresh.
- Building a Custom Dashboard: You can use JavaScript and the REST API to build a custom dashboard for your WordPress site. This could include real-time analytics, recent posts, and other relevant information.
- Developing Interactive Forms: JavaScript can be used to create interactive forms that submit data to the REST API. For example, you could create a comment form that submits comments without reloading the page.
Here is a basic example of how to create a new post using JavaScript and the REST API:
// Define a new post object
const post = {
title: 'Hello World',
content: 'My Post Content.',
status: 'draft'
};
// Call the createPost method from the WordPress instance and log the result
console.log(await wp.createPost(post));
In this example, a new post object is defined with a title, content, and status. The createPost
method is then called to send a POST request to the WordPress REST API, creating a new post with the defined data.
Remember, when working with JavaScript and the REST API, it’s important to consider security and ensure that any sensitive data is properly protected.
Sources:
Using the WordPress REST API | Learn WordPress
Backbone JavaScript Client | REST API Handbook | WordPress Developer Resources
Introduction to WordPress REST API in JavaScript | by Olivia Brown | JavaScript in Plain English
Best Practices for Using JavaScript and the WordPress REST API
When it comes to using JavaScript and the WordPress REST API, there are several best practices that can help ensure your code is efficient, secure, and compatible.
When and Why to Use the REST API in WordPress
The REST API should be used when you need to interact with your WordPress site from outside the WordPress installation itself. This could be for creating interactive websites and apps, or for integrating WordPress with other systems.
However, it’s important to note that you should not feel pressured to use the REST API if your site is already working the way you expect. WordPress already provides a rich set of tools and interfaces for building sites, and the REST API is just another tool in your toolkit.
Tips for Using JavaScript with the REST API
When using JavaScript with the REST API, there are a couple key tips to keep in mind:
- Ensure Compatibility: Make sure your JavaScript code is compatible with the version of the REST API you are using. The REST API is constantly being updated, so it’s important to keep your code up-to-date as well.
- Optimize Performance: Use AJAX calls sparingly and efficiently. Making too many requests to the REST API can slow down your site, so it’s important to only make requests when necessary and to cache results whenever possible.
Handling Authentication and Private Data with the REST API
When using the REST API, it’s crucial to handle authentication and private data securely. The REST API provides public data, which is accessible to any client anonymously, as well as private data available only after authentication.
To ensure that no one can anonymously access site data via the REST API, you can use authentication methods such as OAuth or JWT (JSON Web Tokens). Always make sure to encrypt sensitive data and use secure connections (HTTPS) when interacting with the REST API.
Testing and Debugging with JavaScript and the REST API
Finally, when using JavaScript and the REST API, it’s important to thoroughly test and debug your code. Use tools like Postman for testing API endpoints, and use JavaScript debugging tools (like Chrome Developer Tools) to troubleshoot any issues with your JavaScript code.
Remember, the key to effectively using JavaScript and the WordPress REST API is to always keep the user experience in mind. By following these best practices, you can create interactive and dynamic WordPress sites that are secure, efficient, and user-friendly.
Sources:
The Complete Guide to WordPress REST API Basics
REST API Handbook | WordPress Developer Resources
Introduction to WordPress REST API in JavaScript | by Olivia Brown | JavaScript in Plain English
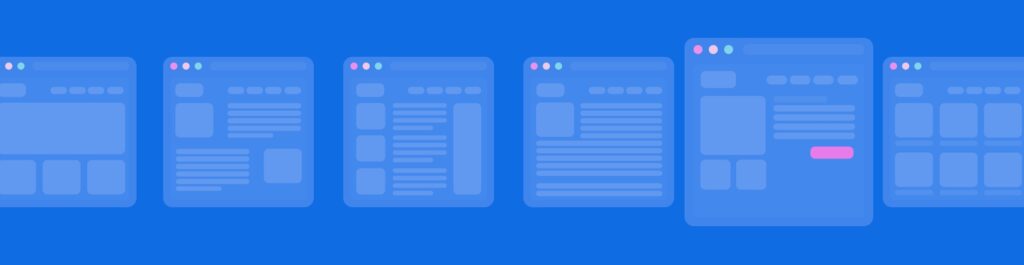
Real-World Applications Using JavaScript and the WordPress REST API
The combination of JavaScript and the WordPress REST API has been used to create a variety of real-world applications. These applications leverage the power of these technologies to provide dynamic, interactive experiences for users. For example:
- E-Commerce Websites: Many e-commerce websites use JavaScript and the WordPress REST API to create dynamic product pages. For example, when a user selects a product variant (like a different color or size), JavaScript can use the REST API to update the product image and price without reloading the page.
- News Websites: News websites often use JavaScript and the REST API to load more articles as the user scrolls down the page. This “infinite scroll” effect can be achieved by using JavaScript to fetch new articles from the REST API whenever the user reaches the bottom of the page.
- Interactive Maps: Websites that feature interactive maps (like real estate websites) can use JavaScript and the REST API to update the map based on user input. For example, if a user selects a different city from a dropdown menu, JavaScript can use the REST API to fetch and display properties in that city.
- Single Page Applications (SPAs): As mentioned earlier, SPAs are web applications that load a single HTML page and dynamically update that page as the user interacts with the app. With JavaScript and the REST API, you can create a SPA within your WordPress site, where each “page” is loaded dynamically without a page refresh.
Benefits of Using JavaScript and the REST API
These examples benefit from using JavaScript and the REST API in several ways:
- Improved User Experience: By allowing for dynamic updates without page reloads, these technologies can provide a smoother, more engaging user experience.
- Increased Efficiency: JavaScript and the REST API can make websites more efficient by only loading or updating the necessary data, rather than reloading the entire page.
- Greater Flexibility: These technologies can enable more complex, interactive features that would be difficult or impossible to achieve with traditional WordPress development.
Real-World Applications
The combination of JavaScript and the WordPress REST API opens up a world of possibilities for developers, allowing them to create more interactive and dynamic websites. This powerful duo is not just a theoretical concept but is actively being used in real-world applications. Here are a few examples:
- YIKES, Inc.: This company has utilized the WordPress REST API to transform websites into data mines. They have built a plugin called WP REST API Controller that enables a UI in the dashboard, allowing users to toggle visibility of custom post types, customize endpoints, and REST API properties. This allows for a more interactive and dynamic website experience.
- Ustwo.com: This website, built by Human Made LTD., is a beautiful example of a full-featured site built on top of the WP REST API. One of the notable features of the site is its fast loading speed and quick page transitions, thanks to the REST API sending JSON data between the server and browser.
- Rachel Baker’s WCUS Demo Theme and Roy Sivan’s Angular WordPress Theme: These are examples of themes built using the REST API, demonstrating how it can be used to construct an entire theme. These themes can be found on Github.
- Kinsta: Kinsta, a managed WordPress hosting provider, uses the WordPress REST API to create a more interactive user experience. Their platform uses the REST API to interface with the WordPress database, fetch data from it, and add data to it, enabling a wide range of applications.
These examples illustrate the power and versatility of using JavaScript and the WordPress REST API together. They show how this combination can be used to create more interactive, dynamic, and user-friendly websites and applications.
Final Thoughts
The combination of JavaScript and the WordPress REST API has revolutionized the way we can use WordPress, transforming it from a simple content management system into a powerful application platform. This powerful duo allows developers to create more interactive, dynamic, and user-friendly websites and applications.
The use of JavaScript with the WordPress REST API allows for the creation of more interactive websites and applications. It enables developers to interact with WordPress data in a structured and predictable way, without needing to reload the page. This not only improves the user experience but also increases the efficiency of the website.
The real-world applications are vast, ranging from e-commerce websites to news websites, interactive maps, and single-page applications. These applications leverage the power of JavaScript and the REST API to provide dynamic, interactive experiences for users, improving user engagement and satisfaction.
However, while the benefits are clear, it’s important to remember the best practices when using JavaScript and the WordPress REST API. Ensuring compatibility, optimizing performance, handling authentication and private data securely, and thorough testing and debugging are all crucial to effectively using these technologies.
The combination of JavaScript and the WordPress REST API opens up a world of possibilities for WordPress developers. Whether you’re looking to create a more interactive website, integrate WordPress with other systems, or even build a full-fledged web application, these technologies provide the tools you need to make it happen.
So, why not give it a try? Start experimenting in your own WordPress projects. You might be surprised at what you can achieve. Remember, the only limit is your imagination and technical expertise. Happy coding!

Before you go, why not stay connected? Subscribe to WordPress Whispers to receive the latest tips, tutorials, and guides on WordPress development. By subscribing, you’ll get expert insights and resources delivered straight to your inbox, helping you stay ahead of the curve in your WordPress development journey. Don’t miss out, subscribe today!
Pingback: Unlocking the Power of WordPress PWAs
Pingback: WordPress vs React - Which Is Right For Your Project?