Table of Contents
Welcome back to the exciting world of WordPress development! If you’re here, you’re probably already familiar with the WordPress REST API, a powerful feature that has revolutionized the way we interact with WordPress. But for those who are new, let’s start with a brief overview.
The WordPress REST API is a feature of WordPress that provides a standardized way of interacting with WordPress sites using HTTP requests. It’s a game-changer because it allows developers to create, read, update, and delete data from their WordPress site using nothing but HTTP requests. This opens up a world of possibilities for integrating WordPress with other services, creating mobile apps, or building interactive JavaScript applications.
Now, let’s talk about custom endpoints. By default, the WordPress REST API provides a set of endpoints for interacting with the core WordPress data types like posts, pages, categories, and more. But what if you want to interact with some custom data type, or you want to create a more complex interaction that isn’t covered by the default endpoints? That’s where custom endpoints come in.
Creating custom endpoints in the WordPress REST API allows you to define your own routes and methods for interacting with your WordPress site. This gives you the power to create highly customized interactions, tailored to your specific needs. Whether you’re building a complex web application or simply want to expose some custom data to the API, custom endpoints are the key to unlocking the full potential of the WordPress REST API.
In this post, we’ll explore the importance of creating custom endpoints, how to create them, and how to use them effectively. We’ll also look at some tools that can make the process easier, and share some best practices for creating and using custom endpoints. So whether you’re a seasoned WordPress developer or just starting out, there’s something here for you.
Here are some resources that you might find helpful as we dive into this topic:
- WordPress REST API Handbook
- Adding Custom Endpoints
- Creating Custom Endpoints in WordPress REST API
- In-Depth Guide in Creating and Fetching Custom WP REST API Endpoints
Let’s get started!
Understanding the Basics of the WordPress REST API
Before we dive into the nitty-gritty of custom endpoints, it’s crucial to understand the basics of the WordPress REST API. So, let’s start with the most fundamental concept: the endpoint.
An endpoint in the context of the WordPress REST API is essentially a URL where your application can send a request to retrieve or manipulate data. It’s like a door to your WordPress site that allows external applications to interact with your content.
The structure of a default WordPress REST API endpoint is straightforward. It typically follows this format: http://yourwebsite.com/wp-json/wp/v2/{resource}
. Here, {resource}
can be posts, pages, categories, and more, depending on what data you want to access.
For example, if you want to retrieve all posts from your WordPress site, you would send a GET request to http://yourwebsite.com/wp-json/wp/v2/posts
. The server would then respond with a JSON object containing the data for all posts.
Data is retrieved and manipulated through these endpoints using standard HTTP methods. For instance, you can use the GET method to retrieve data, POST to create new data, PUT or PATCH to update data, and DELETE to remove data.
Here’s a simple example of how you might retrieve a post with the ID of 1, using the JavaScript fetch()
method:
fetch('http://yourwebsite.com/wp-json/wp/v2/posts/1')
.then(response => response.json())
.then(post => console.log(post));
In this example, we’re using the fetch()
method in JavaScript to send a GET request to the endpoint for the post with the ID of 1. The server responds with the data for that post, which we then log to the console.
Here are additional some resources that you might find helpful as we explore this topic further:
- WordPress REST API Handbook
- WordPress REST API Reference
- Beginner’s Guide to WordPress REST API
- Default REST API Endpoints
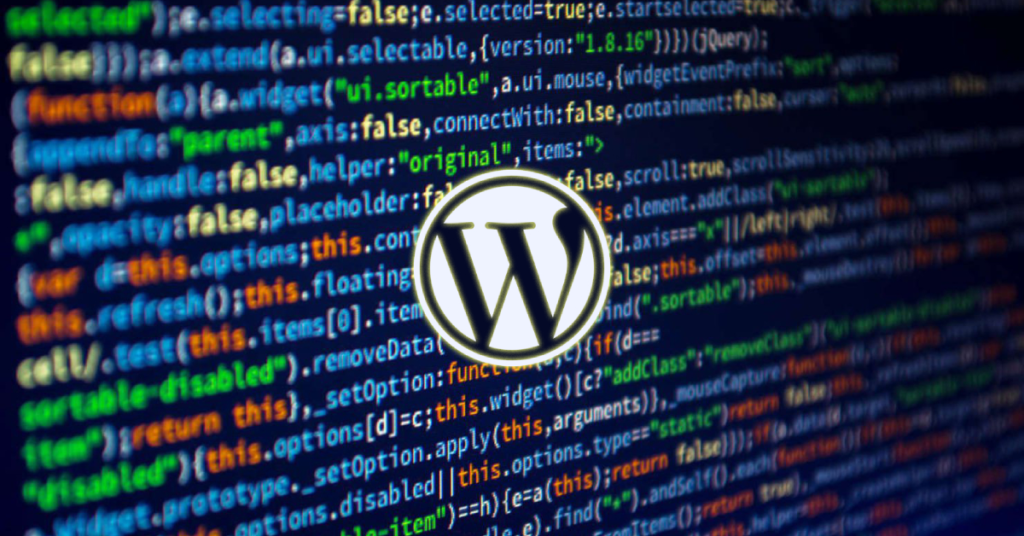
Why Create Custom Endpoints?
As we previously saw, the WordPress REST API comes with a set of built-in endpoints that allow you to interact with posts, pages, categories, and other standard WordPress data types. These default endpoints are incredibly useful for a wide range of applications, but there may be times when they don’t quite meet your needs. That’s where custom endpoints come in.
Imagine you’re building a mobile app for your WooCommerce-powered online store and you want to extract specific data from your WordPress site to use in the app. Or perhaps you’re developing a complex web application that requires interaction with custom post types or complex queries that the default endpoints can’t handle. In these scenarios, the default endpoints might not be sufficient, and creating custom endpoints becomes necessary.
Custom endpoints in the WordPress REST API give you the ability to define your own routes and methods for interacting with your WordPress site. This means you can create highly tailored interactions, giving you more control over how you retrieve and manipulate data.
The benefits of creating custom endpoints are numerous. Here are a few key ones:
Flexibility: Custom endpoints allow you to define exactly what data is returned, how it’s structured, and how it can be manipulated. This means you can tailor the API to fit your specific needs, rather than being constrained by the default endpoints.
Control: With custom endpoints, you have complete control over the data your API exposes. This can be particularly useful if you’re working with sensitive data that you don’t want to expose through the default endpoints.
Integration: Custom endpoints make it easier to integrate your WordPress site with other applications and services. Whether you’re building a mobile app, a web application, or integrating with a third-party service, custom endpoints can make the process smoother and more efficient.
Here are some resources that you might find helpful as we explore this deeper:
- Creating a Custom Endpoint for the REST API
- How to Create Custom Endpoints in WordPress REST API
- Creating Custom Endpoints for the WordPress REST API
- Adding Custom Endpoints
Coming up, we’ll explore how to create these custom endpoints, both by coding them manually and using plugins.
Creating Custom Endpoints – The Traditional Way
The traditional way of creating custom endpoints in the WordPress REST API involves coding in PHP. This method provides a high level of flexibility and control, allowing you to tailor your endpoints to your specific needs. Let’s walk through the process step by step:
Step 1: Planning Your Custom Endpoint
Before you start coding, it’s important to plan out your custom endpoint. What is its purpose? What data will it expose? For example, you might want to create a custom endpoint that retrieves all posts from a specific category, or one that returns user data based on specific criteria. The possibilities are endless, and the endpoint you create should be tailored to your specific needs.
Step 2: Registering a Custom Route
The next step is to register a custom route for your endpoint. This can be done in your theme’s functions.php
file or in a custom plugin file, depending on whether the functionality is theme-specific or should be available regardless of the theme you’re using. It is done using the register_rest_route()
function in WordPress. This function takes three parameters: the namespace (which is typically the name of your plugin or theme), the route (which is the URL where your endpoint will be accessed), and an array of arguments that define how the endpoint behaves.
Here’s an example of how you might register a custom route:
add_action('rest_api_init', function () {
register_rest_route('myplugin/v1', '/author/(?P<id>\d+)', array(
'methods' => 'GET',
'callback' => 'my_awesome_func',
));
});
In this example, myplugin/v1
is the namespace, /author/(?P<id>\d+)
is the route (which includes a regular expression to capture the author ID), and the array specifies that this endpoint responds to GET requests and calls a function named my_awesome_func
when accessed.
Step 3: Defining the Callback Function
The callback function is where the magic happens. This is the function that’s called when your endpoint is accessed, and it’s where you define what data your endpoint returns.
Here’s an example of a simple callback function:
function my_awesome_func($data) {
$posts = get_posts(array(
'author' => $data['id'],
));
if (empty($posts)) {
return null;
}
return $posts[0]->post_title;
}
In this example, the callback function retrieves the first post by the author specified in the URL and returns the post title.
Step 4: Setting Up Parameters
You can also define parameters for your endpoint, which allow users to filter or customize the data that’s returned. This is done using the args
option when registering your route.
Step 5: Testing the Custom Endpoint
Finally, you’ll want to test your custom endpoint to make sure it’s working as expected. You can do this by accessing the URL of your endpoint in your web browser or using a tool like Postman.
Creating custom endpoints in the WordPress REST API using PHP gives you a high level of flexibility and control, allowing you to tailor your endpoints to your specific needs. However, it does require a good understanding of PHP and the WordPress REST API.
Here are some more resources you can explore:
- Adding Custom Endpoints
- Creating a Custom Endpoint for the REST API
- How to Create Custom Endpoints in WordPress REST API
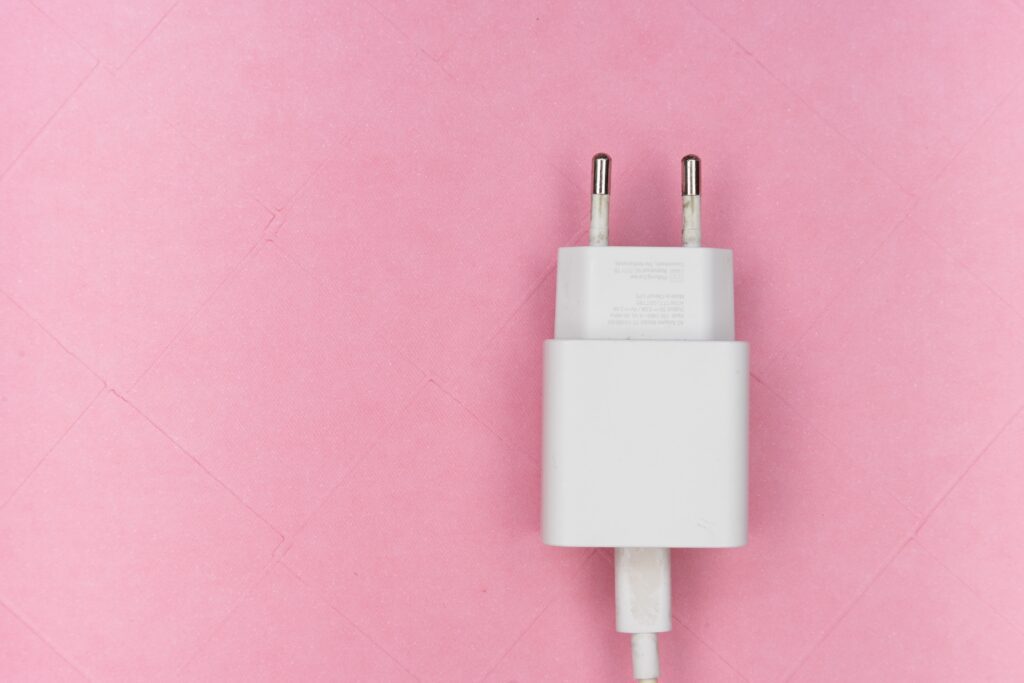
Simplifying Custom Endpoints Creation with Plugins
While the traditional method of creating custom endpoints offers a high level of flexibility and control, it does require a good understanding of PHP and the WordPress REST API. If you’re not comfortable with coding, or if you’re looking for a quicker and easier way to create custom endpoints, there are several plugins that can simplify the process.
Two popular plugins that can help with this are the WP REST API Controller and Custom Post Type UI. These plugins provide a user-friendly interface for creating and managing custom endpoints, without the need for coding.
Here’s a step-by-step guide on how to use these plugins to create custom endpoints:
Step 1: Install and Activate the Plugin
The first step is to install and activate the plugin. You can do this from the Plugins page in your WordPress dashboard.
Step 2: Create a Custom Post Type (Custom Post Type UI)
If you’re using the Custom Post Type UI plugin, you’ll need to create a custom post type. This can be done from the CPT UI menu in your WordPress dashboard.
Step 3: Enable API Access (WP REST API Controller)
If you’re using the WP REST API Controller plugin, you’ll need to enable API access for your custom post type. This can be done from the REST Controller menu in your WordPress dashboard.
Step 4: Define Your Endpoint
Next, you’ll need to define your endpoint. This is done by creating a new route and specifying the method and callback function.
Step 5: Test Your Endpoint
Finally, you’ll want to test your endpoint to make sure it’s working as expected. You can do this by accessing the URL of your endpoint in your web browser or using a tool like Postman.
Compared to the traditional method, using a plugin to create custom endpoints can be much quicker and easier, especially if you’re not comfortable with coding. However, it does offer less flexibility and control, as you’re limited to the features and options provided by the plugin.
Here are some resources on creating custom WordPress REST API endpoints with plugins:
- WP REST API Controller
- Custom Post Type UI
- Creating a Custom Endpoint for the REST API
- How to Create Custom Endpoints in WordPress REST API
Best Practices for Creating Custom WordPress REST API Endpoints
Creating custom endpoints in the WordPress REST API can greatly enhance the functionality of your WordPress site or application. However, it’s important to follow best practices to ensure that your endpoints are secure, performant, and maintainable.
Security Considerations
When creating custom endpoints, security should be a top priority. This includes authentication and permissions.
Authentication ensures that only authorized users can access your endpoints. WordPress provides several methods for authentication, including cookie authentication and application passwords. You can learn more about these methods in the WordPress REST API Handbook.
Permissions determine what an authenticated user can do. For example, you might want to restrict certain endpoints to users with specific roles or capabilities. This can be done using the permission_callback
option when registering your route.
Performance Considerations
Performance is another important consideration when creating custom endpoints. This includes caching and minimizing data transfer.
Caching can greatly improve the performance of your endpoints by storing the results of expensive operations and reusing them for subsequent requests. WordPress provides several functions for caching, including wp_cache_set()
and wp_cache_get()
.
Minimizing data transfer can also improve performance, especially for mobile users or users with slow internet connections. This can be done by only returning the data that’s needed for each request.
Maintainability
Finally, maintainability is crucial for the long-term success of your custom endpoints. This includes code organization and documentation.
Code organization can make your endpoints easier to understand and maintain. This might involve separating your endpoint code into different files or functions based on their functionality.
Documentation can help others (and your future self) understand how your endpoints work and how to use them. This might involve adding comments to your code, or creating separate documentation for your endpoints.
Additional Resources:
- Adding Custom Endpoints
- Creating a Custom Endpoint for the REST API
- How to Create Custom Endpoints in WordPress REST API
- In-Depth Guide in Creating and Fetching Custom WP REST API Endpoints
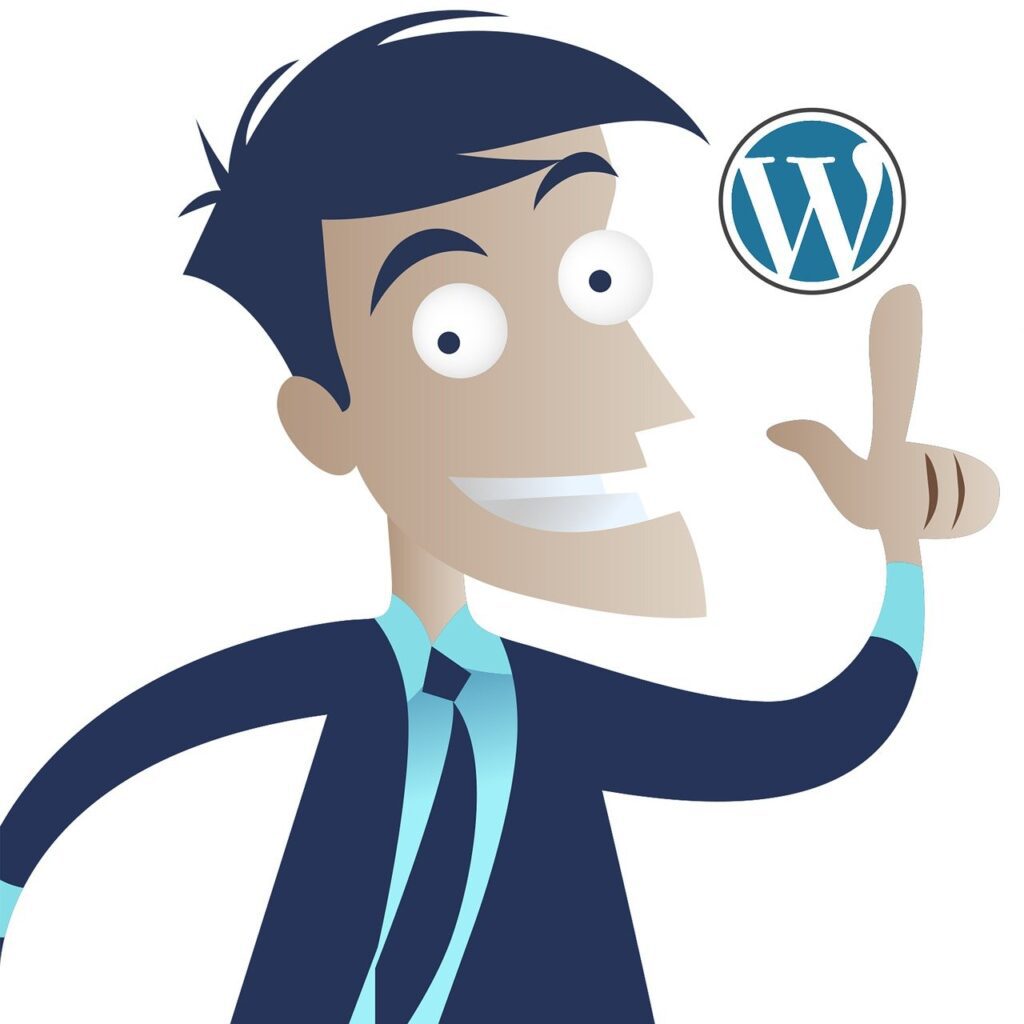
Real-World Use Cases for Custom Endpoints
Custom endpoints in the WordPress REST API can be used in a variety of real-world applications. They can greatly enhance the functionality of your WordPress site or application, providing a more tailored user experience. Here are a few examples:
1. Fetching Posts
One common use of custom endpoints is to fetch posts from your WordPress site. For example, you might create a custom endpoint that retrieves all posts from a specific category or author. This can be useful for creating a custom blog feed or for displaying related posts on a post page.
Here’s an example of how you might create a custom endpoint to fetch posts:
add_action('rest_api_init', function () {
register_rest_route('myplugin/v1', '/posts/(?P<category>\d+)', array(
'methods' => 'GET',
'callback' => 'my_custom_get_posts',
));
});
function my_custom_get_posts($data) {
$posts = get_posts(array(
'category' => $data['category'],
));
if (empty($posts)) {
return null;
}
return $posts;
}
In this example, the custom endpoint retrieves all posts from the category specified in the URL.
2. Creating New Content
Custom endpoints can also be used to create new content on your WordPress site. For example, you might create a custom endpoint that allows users to submit posts or comments from a mobile app or external website.
Here’s an example of how you might create a custom endpoint to create a new post:
add_action('rest_api_init', function () {
register_rest_route('myplugin/v1', '/posts', array(
'methods' => 'POST',
'callback' => 'my_custom_create_post',
));
});
function my_custom_create_post($data) {
$post_id = wp_insert_post(array(
'post_title' => $data['title'],
'post_content' => $data['content'],
'post_status' => 'publish',
));
if (!$post_id) {
return new WP_Error('my_custom_error', 'Could not create post.', array('status' => 500));
}
return array('post_id' => $post_id);
}
In this example, the custom endpoint creates a new post with the title and content specified in the request.
3. Updating Post Meta
Custom endpoints can also be used to update post meta data. For example, you might create a custom endpoint that allows users to update the views count or rating of a post.
Here’s an example of how you might create a custom endpoint to update post meta:
add_action('rest_api_init', function () {
register_rest_route('myplugin/v1', '/posts/(?P<id>\d+)/meta', array(
'methods' => 'PUT',
'callback' => 'my_custom_update_post_meta',
));
});
function my_custom_update_post_meta($data) {
$updated = update_post_meta($data['id'], $data['key'], $data['value']);
if (!$updated) {
return new WP_Error('my_custom_error', 'Could not update post meta.', array('status' => 500));
}
return array('updated' => true);
}
In this example, the custom endpoint updates the post meta for the post and meta key specified in the URL with the value specified in the request.
These are just a few examples of how custom endpoints can be used in real-world applications. The possibilities are virtually endless, and with a bit of creativity and technical know-how, you can leverage the power of custom endpoints to create a more dynamic and interactive user experience on your WordPress site or application.
Additional Resources:
- WordPress REST API: Adding Custom Endpoints
- How to Add Custom Endpoints to WordPress API
- Adding Custom Endpoints: Extra Touches
- How to Use WordPress REST API
Troubleshooting Common Problems with Custom Endpoints
Creating custom endpoints in the WordPress REST API can sometimes be a challenging task, especially if you’re new to the process. You might encounter a few common issues along the way. But don’t worry, we’ve got you covered. Here are some common issues and their solutions:
1. 404 Error: Endpoint Not Found
One of the most common issues you might encounter is a 404 error indicating that your custom endpoint was not found. This can happen if the route for your endpoint is not correctly registered, or if there’s a typo in the URL for your endpoint.
To resolve this issue, double-check the code where you registered your route. Make sure the namespace, route, and callback function are correctly defined. Also, make sure you’re using the correct URL to access your endpoint.
2. 403 Error: Forbidden
Another common issue is a 403 error indicating that you’re forbidden from accessing your endpoint. This can happen if the user trying to access the endpoint does not have the necessary permissions.
To resolve this issue, check the permission_callback
option in your route registration. Make sure it’s correctly set up to grant access to the appropriate users.
3. No Data Returned
You might also encounter an issue where your endpoint does not return any data. This can happen if there’s an error in your callback function, or if the data you’re trying to retrieve does not exist.
To resolve this issue, check your callback function to make sure it’s correctly retrieving and returning the data. Also, check the data source (e.g., the database) to make sure the data you’re trying to retrieve actually exists.
4. Slow Performance
If your endpoint is slow to respond, it might be due to inefficient data retrieval or processing. This can happen if you’re retrieving a large amount of data, or if you’re performing complex operations on the data.
To resolve this issue, consider implementing caching to store the results of expensive operations. Also, consider optimizing your data retrieval and processing to only retrieve and process the data that’s actually needed.
Additional Troubleshooting Help:
- Why is my custom API endpoint not working?
- WP REST API custom post endpoint not working – 404 error
- WordPress REST API custom endpoints not working with ID parameters
- Common WordPress REST API Errors
- Verify WordPress REST API is Active
Final Thoughts
In this post, we’ve explored the power and flexibility of the WordPress REST API, particularly the ability to create custom endpoints. Whether you’re a seasoned developer or just a WordPress enthusiast, understanding how to create and use custom endpoints can greatly enhance the functionality and user experience of your WordPress site or application.
We’ve discussed the traditional method of creating custom endpoints through coding in PHP, as well as the simplified process using plugins like WP REST API Controller and Custom Post Type UI. We’ve also delved into best practices for creating custom endpoints, including security, performance, and maintainability considerations, and provided real-world examples of how custom endpoints can be used.
Creating custom endpoints might seem daunting at first, but with a bit of practice and experimentation, you’ll soon discover the immense potential they offer. Whether you choose to code your own endpoints or use a plugin, the important thing is to start experimenting and see what you can create.
As a final note, we’d like to invite you to subscribe to WordPress Whispers. As a subscriber, you’ll not only get the latest tips and tutorials on WordPress development, but you’ll also get a free copy of our ebook, “Headless WordPress: A New Era of Web Development”, normally priced at $49.99. This comprehensive guide will take you further into the world of headless WordPress development, providing you with the knowledge and skills to create even more powerful and dynamic WordPress applications.
So why wait? Start your journey into the world of custom endpoints and headless WordPress development today. Subscribe to WordPress Whispers and get your free ebook now!
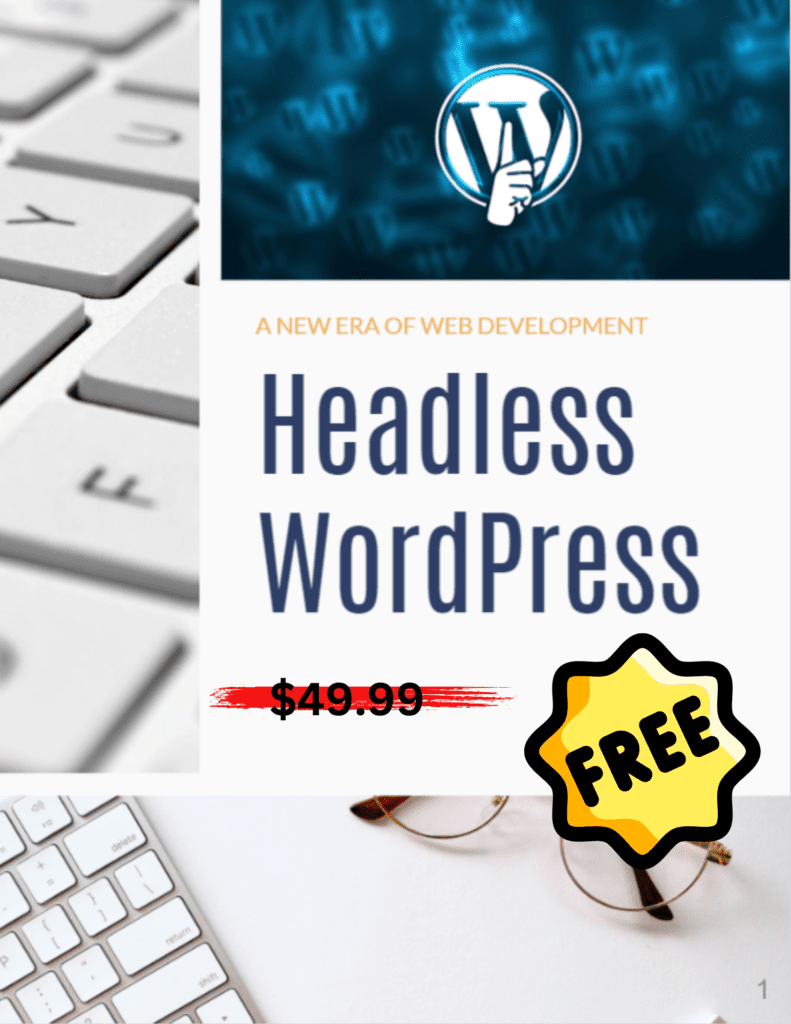
Pingback: Mastering CORS Integration in WordPress | WordPress Whispers
Pingback: Customizing WooCommerce API Responses | WordPress Whispers
Pingback: Setting Up a Headless WordPress Environment with React