Table of Contents
Welcome to WordPress Whispers, your go-to resource for all things WordPress and JavaScript! Today, we’re discussing a topic that’s crucial for any web developer working with WordPress: the WordPress REST API and the importance of user authentication in web applications.
The WordPress REST API is a feature of WordPress that provides a way for applications to interact with your WordPress site. It allows developers to send and receive data using HTTP requests, making it possible to manage and retrieve data from your site using JavaScript, mobile apps, or other external applications.
But why is this important? Well, the WordPress REST API opens up a world of possibilities for enhancing your WordPress site’s interactivity and functionality. However, with great power comes great responsibility. This is where user authentication comes into play.
User authentication is a process that verifies the identity of a user who is trying to access a system. In the context of the WordPress REST API, user authentication is crucial for ensuring that only authorized users can perform certain actions, such as creating, updating, or deleting data. This not only helps to keep your site secure but also ensures that each user has a personalized and seamless experience.
In the following sections, we’ll delve deeper into what user authentication is, why it’s important in the context of the WordPress REST API, and the different methods of user authentication available. We’ll also provide step-by-step guides and code examples for implementing each method, so you can choose the one that best suits your needs.
So let’s dive in and uncover the potential of the WordPress REST API together!
Here are some resources you may find helpful as we go along:
WordPress REST API Handbook
WordPress REST API: An Introduction
Understanding Authentication
What Is User Authentication?
User authentication, in its simplest form, is the process of verifying the identity of a user who attempts to access a system. It’s like a digital handshake between the user and the system, ensuring that the user is who they claim to be. This process is crucial in maintaining the security and integrity of any web application, including those built with the WordPress REST API.
In the context of the WordPress REST API, user authentication plays a pivotal role. It ensures that only authorized users can perform certain actions, such as creating, updating, or deleting data. This not only helps to keep your WordPress site secure but also ensures that each user has a personalized and seamless experience.
Now, let’s delve into the different methods of user authentication available in the WordPress REST API. There are four primary methods: Cookie Authentication, Basic Authentication, OAuth Authentication, and JWT Authentication. Each of these methods has its own strengths and weaknesses, and the best one for your application will depend on your specific needs and circumstances.
Coming up, we’ll explore each of these methods in detail, providing you with the knowledge you need to make an informed decision about which one is right for your WordPress project.
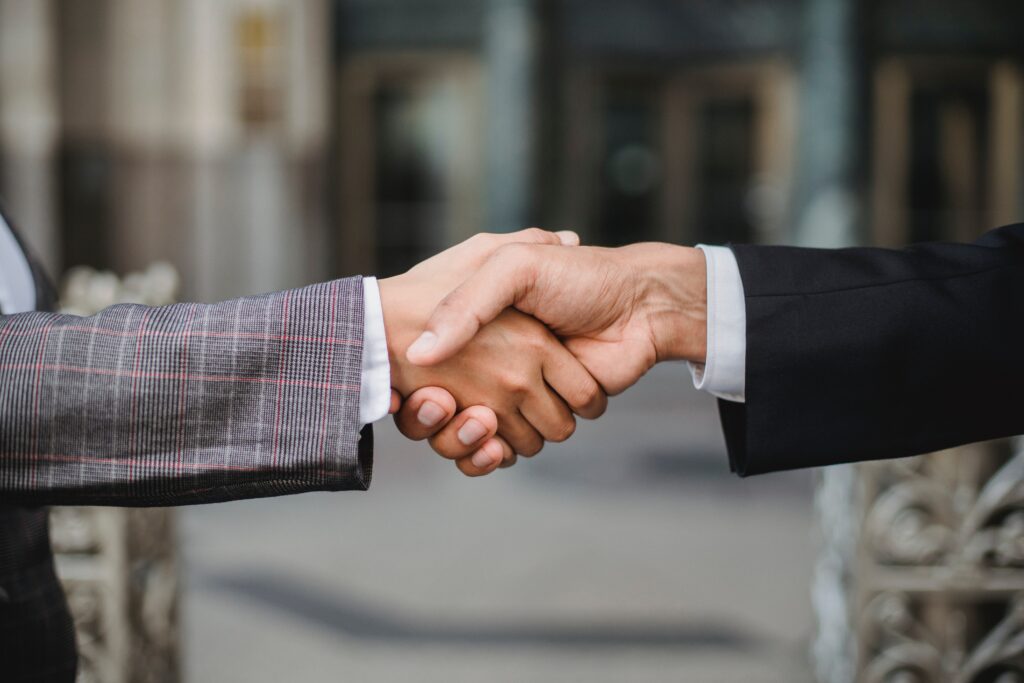
Brief Overview of Various Authentication Methods
Let’s start our journey into the world of user authentication in the WordPress REST API with an overview of the different methods available. These methods include Cookie Authentication, Basic Authentication, OAuth Authentication, and JWT Authentication.
Cookie Authentication is the standard authentication method included with WordPress. When a user logs in to the WordPress dashboard, they are authenticated by means of cookies. This method is ideal for plugins and themes that need to make authenticated requests to the WordPress REST API from the browser.
Basic Authentication is a simple method that requires a username and password with each request. This method is not secure unless used in conjunction with other security measures, such as HTTPS, as the username and password are transmitted in clear text.
OAuth Authentication is a more secure method that allows applications to authenticate without storing user credentials. Instead, it uses tokens that can be revoked and regenerated as needed. This method is ideal for third-party applications that need to access the WordPress REST API on behalf of users.
JWT Authentication (JSON Web Tokens) is a method that uses JSON Web Tokens to authenticate users. This method is ideal for single page applications (SPAs) where the token can be stored in the browser and used to make authenticated requests.
Now, let’s dive deeper into the first method: Cookie Authentication.
Cookie Authentication
Cookie Authentication is the built-in method that WordPress uses to authenticate a user. When a user logs into their WordPress account, WordPress generates a unique identifier known as a cookie. This cookie is then stored in the user’s web browser. When the user makes subsequent requests to the server, the browser automatically includes this cookie. The server can then use this cookie to verify the identity of the user, thus authenticating them.
In the context of the WordPress REST API, Cookie Authentication is especially useful for plugins and themes that need to make authenticated requests from the client side. This is because the browser automatically includes the cookie with each request, so the user doesn’t have to manually input their credentials each time.
Here’s a more detailed step-by-step guide on how to implement Cookie Authentication:
User Login: The user logs into their WordPress account through the standard WordPress login page. Upon successful login, WordPress generates an authentication cookie for the user.
Cookie Storage: The cookie is then stored in the user’s web browser. The exact location of storage can vary depending on the browser, but generally, the cookie is stored in a dedicated location within the browser’s file system.
Authenticated Requests: The cookie can now be used to authenticate requests to the WordPress REST API. When a request is made, the browser automatically includes the cookie in the request header. This allows the server to recognize the user and authenticate the request.
Here’s an example of how to make an authenticated request using JavaScript and jQuery:
jQuery.ajax({
url: 'https://yourwebsite.com/wp-json/wp/v2/posts',
method: 'POST',
beforeSend: function (xhr) {
xhr.setRequestHeader('X-WP-Nonce', wpApiSettings.nonce);
},
data:{
'title': 'Your Post Title',
'content': 'Your post content',
'status': 'publish'
}
}).done(function (response) {
console.log(response);
});
In this code:
jQuery.ajax()
is a function that makes an HTTP request. It takes an object as an argument, which contains the details of the request.url
is the endpoint of the WordPress REST API where you want to send the request. Replace ‘https://yourwebsite.com’ with your actual website URL.method
is the HTTP method to use for the request. In this case, ‘POST’ (HTTP POST request) is used to create a new post (WordPress Post Data Type).beforeSend
is a function that is called before the request is sent. It is used here to set the ‘X-WP-Nonce’ header, which is required for Cookie Authentication.xhr.setRequestHeader('X-WP-Nonce', wpApiSettings.nonce)
sets the ‘X-WP-Nonce’ header to the nonce associated with the logged-in user. The nonce is a “number used once” that WordPress generates and associates with the logged-in user. It is used to prevent CSRF attacks.data
is the data to send with the request. In this case, it’s the details of the post to create.done
is a function that is called when the request is successful. It logs the response to the console.
This code should be added to a JavaScript file that is enqueued in your WordPress theme or plugin.
Additional Resources:
WordPress REST API Handbook: Cookie Authentication
A Comprehensive Guide to Authenticating to APIs
Basic Authentication
Basic Authentication is a straightforward and widely used method for user authentication. It operates by sending the user’s credentials (username and password) with each HTTP request. These credentials are encoded with Base64 and included in the Authorization header of the request.
While Basic Authentication is simple to implement, it comes with a significant security concern: the user’s credentials are sent in clear text (albeit Base64-encoded) with each request. This means that if the connection is not secure, the credentials could be intercepted by malicious parties. Therefore, it’s crucial to use Basic Authentication in conjunction with HTTPS, which encrypts the data sent between the client and the server, providing a secure communication channel.
Here’s a detailed step-by-step guide on how to implement Basic Authentication:
Install and activate the Basic Auth plugin: WordPress does not support Basic Authentication out of the box. However, you can add support for it by installing and activating the Basic Auth plugin. This plugin is available on the WordPress GitHub repository. To install it, you need to download the plugin, upload it to your WordPress plugins directory, and then activate it from the WordPress admin dashboard.
Make an authenticated request: Once the plugin is activated, you can make authenticated requests to the WordPress REST API. Here’s an example of how to do this using JavaScript’s Fetch API:
const username = 'your_username';
const password = 'your_password';
const url = 'https://yourwebsite.com/wp-json/wp/v2/posts';
fetch(url, {
method: 'POST',
headers: {
'Authorization': 'Basic ' + btoa(username + ':' + password),
'Content-Type': 'application/json'
},
body: JSON.stringify({
'title': 'Your Post Title',
'content': 'Your post content',
'status': 'publish'
})
})
.then(response => response.json())
.then(data => console.log(data))
.catch((error) => console.error('Error:', error));
In this code:
btoa(username + ':' + password)
is a JavaScript function that encodes the username and password in Base64. Thebtoa
function takes a string and returns a Base64-encoded string.'Authorization': 'Basic ' + btoa(username + ':' + password)
sets the Authorization header to ‘Basic ‘ followed by the Base64-encoded username and password. This is how the credentials are sent with each request.JSON.stringify({ ... })
is a JavaScript function that converts a JavaScript object into a JSON string. This is necessary because the body of the request needs to be a string, not an object.
Remember to replace ‘your_username’, ‘your_password’, and ‘https://yourwebsite.com’ with your actual username, password, and website URL.
For further reading, you might find these resources helpful:
WordPress REST API Handbook: Basic Authentication
A Comprehensive Guide to Authenticating to APIs
Understanding Authentication, Authorization, and Encryption
OAuth (Open Authorization) Authentication
OAuth (Open Authorization) is an open standard for access delegation. It’s a more complex method of authentication, but it provides a secure and efficient way for users to authorize third-party applications to access their data without sharing their credentials (username and password).
In the context of the WordPress REST API, OAuth Authentication allows third-party applications to make authenticated requests on behalf of the user. This is particularly useful for applications that need to interact with the WordPress REST API on a user’s behalf, such as social media apps that post to a user’s WordPress blog.
Here’s a detailed step-by-step guide on how to implement OAuth Authentication:
Install and activate the OAuth plugin: Like Basic Authentication, WordPress does not support OAuth Authentication out of the box. However, you can add support for it by installing and activating the OAuth plugin. You can download the plugin from the WordPress GitHub repository.
Register a new application: Once the plugin is activated, you need to register a new application. This can be done from the WordPress admin dashboard. When you register an application, you will receive a client key and client secret, which are used to identify the application in the OAuth flow.
Authorize the application: The user needs to authorize the application to access their data. This is done through an authorization page, where the user can see what data the application wants to access and either approve or deny the request.
Obtain an access token: If the user approves the request, the application will receive an authorization code. The application can exchange this code for an access token by making a POST request to the token endpoint.
Make authenticated requests: The application can now use the access token to make authenticated requests to the WordPress REST API on behalf of the user.
Here’s an example of how to obtain an access token using JavaScript:
const clientKey = 'your_client_key';
const clientSecret = 'your_client_secret';
const authorizationCode = 'your_authorization_code';
const url = 'https://yourwebsite.com/oauth1/access';
fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
body: `client_id=${clientKey}&client_secret=${clientSecret}&code=${authorizationCode}`
})
.then(response => response.json())
.then(data => console.log(data))
.catch((error) => console.error('Error:', error));
In this code:
client_id=${clientKey}&client_secret=${clientSecret}&code=${authorizationCode}
is the data to send with the request. It includes the client key, client secret, and authorization code.fetch(url, { ... })
makes a POST request to the token endpoint to exchange the authorization code for an access token.
Remember to replace ‘your_client_key’, ‘your_client_secret’, ‘your_authorization_code’, and ‘https://yourwebsite.com’ with your actual client key, client secret, authorization code, and website URL.
Additional resources you may find helpful:
WordPress REST API Handbook: OAuth Authentication
A Comprehensive Guide to Authenticating to APIs
Understanding Authentication, Authorization, and Encryption
JWT (JSON Web Tokens) Authentication
JWT, or JSON Web Tokens, is another popular method of authentication that is often used in the context of APIs. JWTs provide a way of transmitting information between parties as a JSON object in a safe and secure manner. This information can be verified and trusted because it is digitally signed.
In the context of the WordPress REST API, JWT Authentication allows the server to verify the identity of the client by looking at the token they provide. This is particularly useful for applications that need to make authenticated requests to the WordPress REST API, such as mobile apps or single-page applications.
Here’s a detailed step-by-step guide on how to implement JWT Authentication:
Install and activate the JWT Authentication plugin: WordPress does not support JWT Authentication out of the box. However, you can add support for it by installing and activating the JWT Authentication plugin. You can download the plugin from the WordPress plugin repository.
Generate a secret key: Once the plugin is activated, you need to generate a secret key. This key is used to sign the tokens and can be any string of characters. You should store this key in your wp-config.php file as a constant.
Authenticate the user: To authenticate a user, you need to make a POST request to the /jwt-auth/v1/token endpoint with the user’s username and password in the body of the request. If the credentials are correct, the server will return a token.
Make authenticated requests: To make an authenticated request, you need to include the token in the Authorization header of the request. The header should look like this: Authorization: Bearer your-token.
Here’s an example of how to authenticate a user and make an authenticated request using JavaScript:
const username = 'your_username';
const password = 'your_password';
const url = 'https://yourwebsite.com/jwt-auth/v1/token';
fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ username, password })
})
.then(response => response.json())
.then(data => {
const token = data.token;
// Make an authenticated request
fetch('https://yourwebsite.com/wp-json/wp/v2/posts', {
headers: {
'Authorization': `Bearer ${token}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch((error) => console.error('Error:', error));
})
.catch((error) => console.error('Error:', error));
In this code:
JSON.stringify({ username, password })
is the data to send with the request. It includes the username and password.fetch(url, { ... })
makes a POST request to the token endpoint to authenticate the user and get a token.fetch('https://yourwebsite.com/wp-json/wp/v2/posts', { ... })
makes an authenticated GET request to the posts endpoint of the WordPress REST API.
Remember to replace ‘your_username’, ‘your_password’, and ‘https://yourwebsite.com’ with your actual username, password, and website URL.
Additional Helpful Resources:
WordPress REST API Handbook: JWT Authentication
A Comprehensive Guide to Authenticating to APIs
Understanding Authentication, Authorization, and Encryption
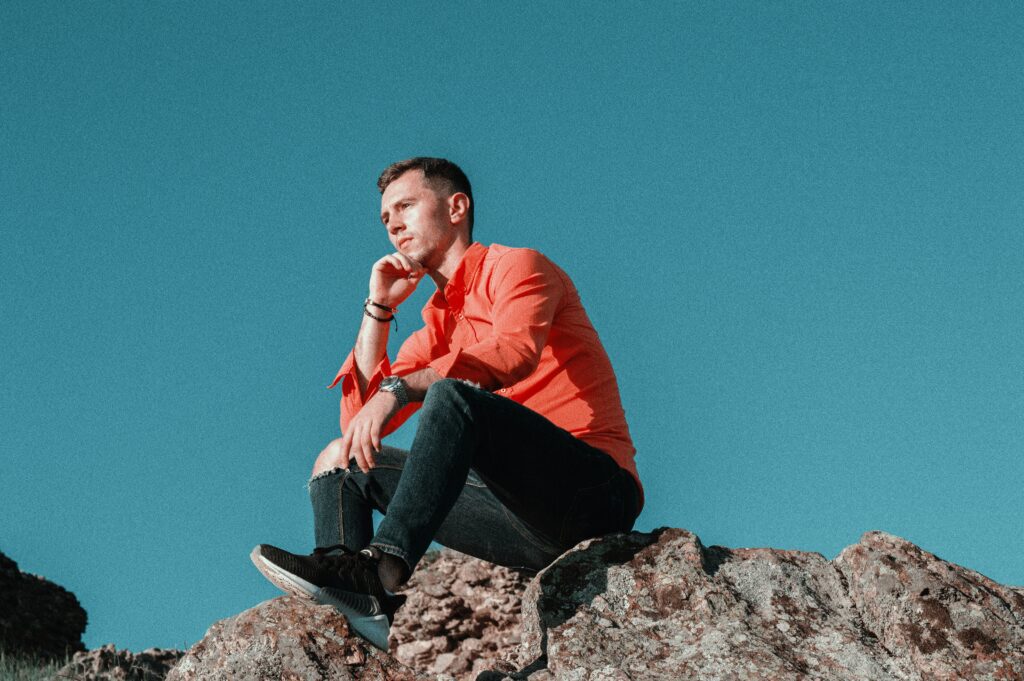
Choosing An Authentication Method
Choosing the right authentication method for your WordPress REST API is a crucial decision that can impact the security, ease of implementation, and use cases of your application. Here are some factors to consider and a comparison of the different methods we’ve discussed:
Factors To Consider
Security: The security of your application should be your top priority. All four methods (Cookie, Basic, OAuth, and JWT Authentication) provide a certain level of security, but they each have their strengths and weaknesses. For example, Basic Authentication is simple and easy to implement, but it’s not the most secure method because it involves sending the username and password with each request.
Ease of Implementation: Some methods are easier to implement than others. Basic Authentication and Cookie Authentication are relatively straightforward, while OAuth and JWT Authentication are more complex but offer more flexibility and security.
Use Cases: The best method for you depends on your specific use cases. For example, if you’re building a simple application that only needs to authenticate users, Basic Authentication might be sufficient. But if you’re building a more complex application that needs to authorize third-party applications to access user data, you might want to consider OAuth or JWT Authentication.
Comparison of Different Methods
Cookie Authentication: This is the simplest method and is built into WordPress by default. It’s easy to implement but is only suitable for logged-in users and is less secure than other methods.
Basic Authentication: This method involves sending the username and password with each request. It’s easy to implement but is not the most secure method. It’s suitable for testing and development but not recommended for production environments.
OAuth Authentication: This is a more secure and flexible method that allows third-party applications to make authenticated requests on behalf of the user. It’s more complex to implement but is suitable for production environments and applications that need to authorize third-party applications.
JWT Authentication: This method involves generating a token that can be used to authenticate requests. It’s secure and flexible, and the token can be stored and reused, reducing the need for repeated logins. It’s more complex to implement but is suitable for production environments and applications that need to authenticate requests over a long period.
Remember, there’s no one-size-fits-all solution. The best method for you depends on your specific needs and use cases. It’s always a good idea to consult with a security expert or do further research before making a decision.
Additional Resources:
WordPress REST API Handbook: Authentication
A Comprehensive Guide to Authenticating to APIs
Understanding Authentication, Authorization, and Encryption
Next, we’ll discuss best practices for user authentication.
Best Practices for User Authentication
When it comes to user authentication in the WordPress REST API, there are several best practices to follow. These practices ensure that your application is secure, performs well, and is easy to maintain. Let’s delve into these considerations:
Security Considerations
Use HTTPS: Always use HTTPS instead of HTTP to ensure that all communication between your application and the server is encrypted. This is especially important when you’re sending sensitive data like usernames and passwords.
Validate and Sanitize Data: Always validate and sanitize user input to protect your application from common security vulnerabilities like SQL injection and cross-site scripting (XSS). WordPress provides several functions to help with this, such as sanitize_text_field()
and wp_kses()
.
Limit Failed Login Attempts: To protect against brute force attacks, consider limiting the number of failed login attempts from a single IP address. There are several plugins available that can help with this.
Performance Considerations
Use Tokens Instead of Cookies for Mobile Apps: If you’re building a mobile app, consider using a method like JWT Authentication that uses tokens instead of cookies. Tokens are more lightweight and can be stored and reused, reducing the need for repeated logins.
Maintainability
Use Plugins for Easier Implementation: WordPress does not support methods like Basic, OAuth, and JWT Authentication out of the box. However, there are several plugins available that add support for these methods and make them easier to implement.
Remember, these are just best practices and may not apply to every situation. Always consider your specific needs and use cases when deciding how to implement user authentication in your application.
For further reading, you might find these resources helpful:
WordPress REST API Handbook: Authentication
A Comprehensive Guide to Authenticating to APIs
Understanding Authentication, Authorization, and Encryption
Next, we’ll discuss common issues that might arise when implementing user authentication and how to resolve them.
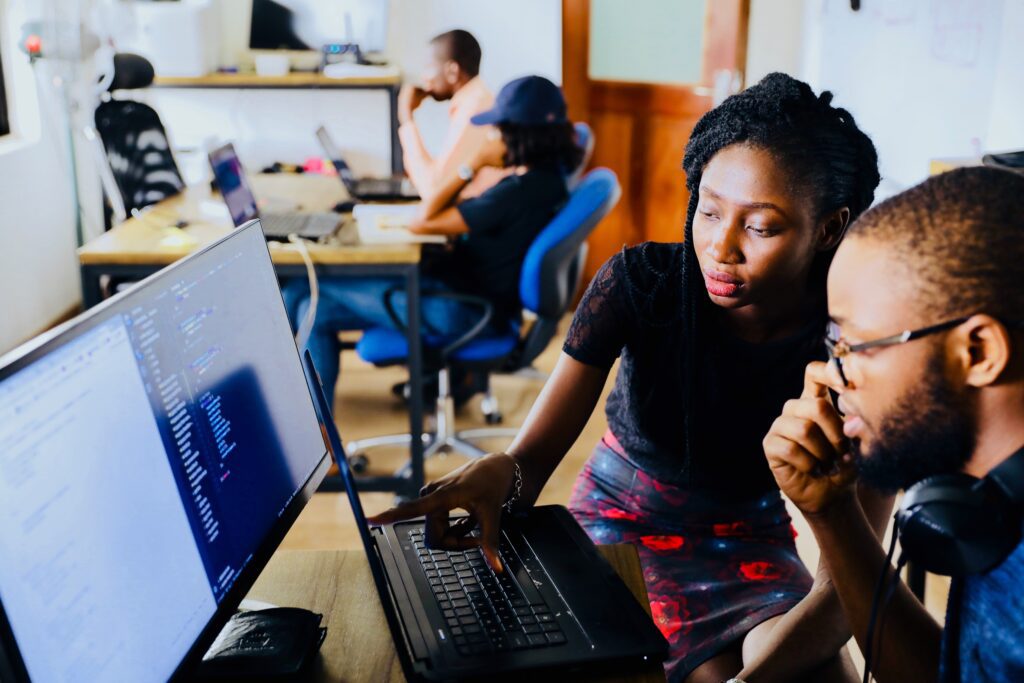
Troubleshooting Common Issues
Implementing user authentication in the WordPress REST API can sometimes be a challenging task. However, understanding the common issues that might arise and knowing how to resolve them can make the process smoother. Here are some common issues and their solutions:
Invalid Credentials
This is one of the most common issues. If the username or password is incorrect, the server will return a 401 Unauthorized response. Make sure you’re using the correct credentials and that the user exists in the WordPress database.
Missing or Invalid Token
If you’re using a method like OAuth or JWT Authentication, you need to include a valid token in the Authorization header of the request. If the token is missing or invalid, the server will return a 403 Forbidden response. Make sure you’re including the token in the correct format (e.g., Authorization: Bearer your-token).
CORS Issues
Cross-Origin Resource Sharing (CORS) is a mechanism that allows many resources (e.g., fonts, JavaScript, etc.) on a web page to be requested from another domain outside the domain from which the resource originated. If you’re making requests from a different domain, you might encounter CORS issues. To resolve this, you can add the appropriate CORS headers to the server’s response. There are several plugins available that can help with this.
Plugin Conflicts
Sometimes, other plugins can interfere with the authentication process. If you’re experiencing issues, try disabling other plugins to see if the issue is resolved. If it is, you can enable the plugins one by one to identify which one is causing the conflict.
Incorrect Server Configuration
If the server is not correctly configured, it might not support the authentication method you’re using. For example, some servers do not support the Authorization header used in Basic, OAuth, and JWT Authentication. Check the server’s documentation or consult with a server administrator to ensure it’s correctly configured.
Remember, these are just common issues, and the specific issues you encounter might be different. Always test your application thoroughly and consult the documentation or seek help from the community if you’re stuck.
Final Thoughts
As we wrap up our discussion on user authentication in the WordPress REST API, let’s take a moment to recap the importance and benefits of this crucial aspect of web development.
User authentication is the cornerstone of secure web applications. It ensures that only authorized users can access certain parts of your application, protecting sensitive data from unauthorized access. In the context of the WordPress REST API, user authentication allows you to control who can create, read, update, and delete data, providing a layer of security and control.
We’ve explored four different methods of user authentication: Cookie Authentication, Basic Authentication, OAuth Authentication, and JWT Authentication. Each method has its strengths and weaknesses, and the best method for you depends on your specific needs and use cases.
Implementing user authentication can be a complex task, but the benefits are well worth the effort. Not only does it improve the security of your application, but it also enhances the user experience by allowing users to interact with your application in more meaningful ways.
We encourage you to experiment with implementing different authentication methods in your WordPress REST API. Don’t be afraid to try new things and learn from your mistakes. Remember, the best way to learn is by doing.
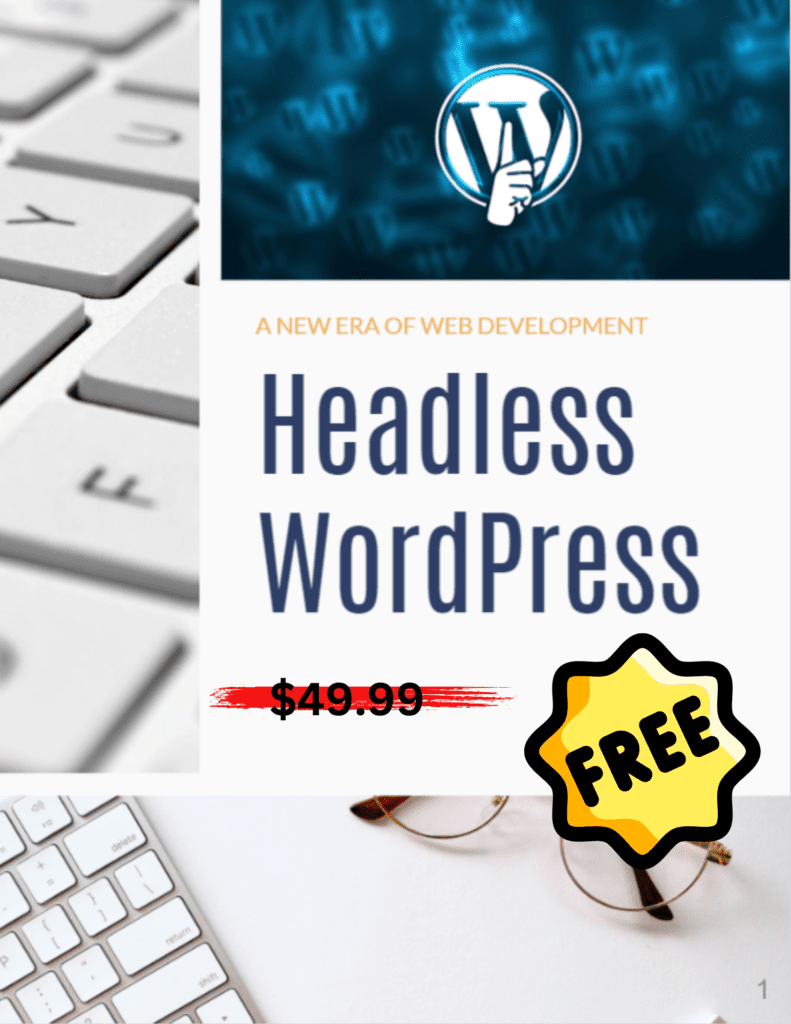
Finally, if you found this guide helpful and want to learn more about WordPress development, consider subscribing to WordPress Whispers. As a subscriber, you’ll get access to a wealth of resources, including our free ebook on Headless WordPress. Join our community of WordPress enthusiasts and experts and take your WordPress skills to the next level.
Thank you for reading, and happy coding!