Table of Contents
The ability to adapt and customize your online store is not just a luxury but a necessity in the evolving landscape of e-commerce. The WooCommerce API, powered by WordPress and WooCommerce, has become a popular choice for these platforms, thanks to its flexibility and extensive customization options. With nearly 4.5 million websites using WooCommerce and its commanding market share in the e-commerce domain, understanding and leveraging the WooCommerce API is crucial for any store owner aiming for success and scalability.
The WooCommerce API, an extension of the WordPress REST API, is tailored specifically for WooCommerce stores. It allows developers and store owners to interact with and manipulate store data such as products, orders, and customers. This API’s powerful capabilities enable integration with external systems like inventory management software or Customer Relationship Management (CRM) systems, significantly streamlining operations.
However, the default API responses may not always fit your specific business needs or desired workflows. This is where customizing WooCommerce API responses becomes essential. By tailoring these responses, you can enhance your store’s efficiency, provide personalized experiences, and ensure seamless integration with other systems. Customizing API responses can range from modifying product details to creating custom integration with a CRM system, automating inventory management, or even building a personalized mobile app for your store.
This article is dedicated to providing an in-depth guide on customizing WooCommerce API responses. We’ll delve into the different ways you can modify these responses, the advantages of doing so, and practical examples with code examples to help you implement these customizations in real-life. Whether you are a seasoned developer or a store owner with access to technical resources, my goal is to to equip you with the knowledge and tools to customize your WooCommerce store effectively.
Stay tuned as we delve into the techniques and methods for transforming your WooCommerce store into a more efficient, responsive, and customized e-commerce platform.
Additional Resources:
WooCommerce REST API Documentation
Insight on WooCommerce REST API Functionality
Trends and Statistics in WooCommerce

Understanding the Basics of WooCommerce REST API Responses
The WooCommerce API plays a crucial role in managing and customizing your online store. Understanding the structure of its responses is key to effectively utilizing this powerful tool.
Typical Structure of WooCommerce API Responses
API responses in WooCommerce are generally formatted in JSON, providing a structured, readable, and easy-to-parse data format. Key components of these responses include:
- Data Fields: These include the essential details related to the request, such as product information, order details, customer data, etc. Each data type has specific fields that are returned in the response.
- Pagination Information: For requests that can return multiple items (like products or orders), pagination information is included, showing links to the next, last, first, and previous pages of results. This helps in navigating through large sets of data effectively.
- Status Codes and Messages: Each API response comes with a status code indicating the success or failure of the request. These codes are critical for error handling and understanding the API’s behavior.
Authentication and Security
The WooCommerce API uses keys for authentication, ensuring secure access to your store’s data. You’ll need to generate API keys from the WooCommerce settings, which are then used to authenticate API requests. These keys are typically included in the request header. The API supports different authentication methods like Basic Auth and OAuth, depending on whether your site is served over HTTPS or HTTP.
Making API Requests
Interacting with the WooCommerce REST API involves sending HTTP requests to specific endpoints of your WooCommerce store. Each endpoint corresponds to different functionalities like retrieving product details or updating order status. The requests are typically in the form of GET, POST, PUT, or DELETE, depending on the action you want to perform.
Common Challenges and Solutions
While the WooCommerce API is powerful, working with it can present challenges such as:
- Security Management: Ensuring the protection of sensitive data like customer information and payment details is crucial. Implementing robust authentication and authorization is key to safeguarding this data.
- Technical Expertise: Effective use of the API requires a good understanding of web technologies and API interactions. For many businesses, this might necessitate hiring experienced developers or seeking professional assistance.
- Performance Impact: The way your API is set up can significantly affect the user experience on your site. Factors like server load and network latency need careful consideration to ensure smooth operation.
Tools and Resources
To work with the WooCommerce API, tools like Postman and Insomnia can be incredibly helpful. They allow you to test and debug your API requests and responses easily. Moreover, the WooCommerce community provides extensive documentation and support, making it easier to navigate the complexities of API integration.
As we delve deeper, we will uncover the immense potential of the WooCommerce API to transform and elevate your online store. For further insights and detailed guides, exploring the official WooCommerce REST API documentation can be extremely beneficial.
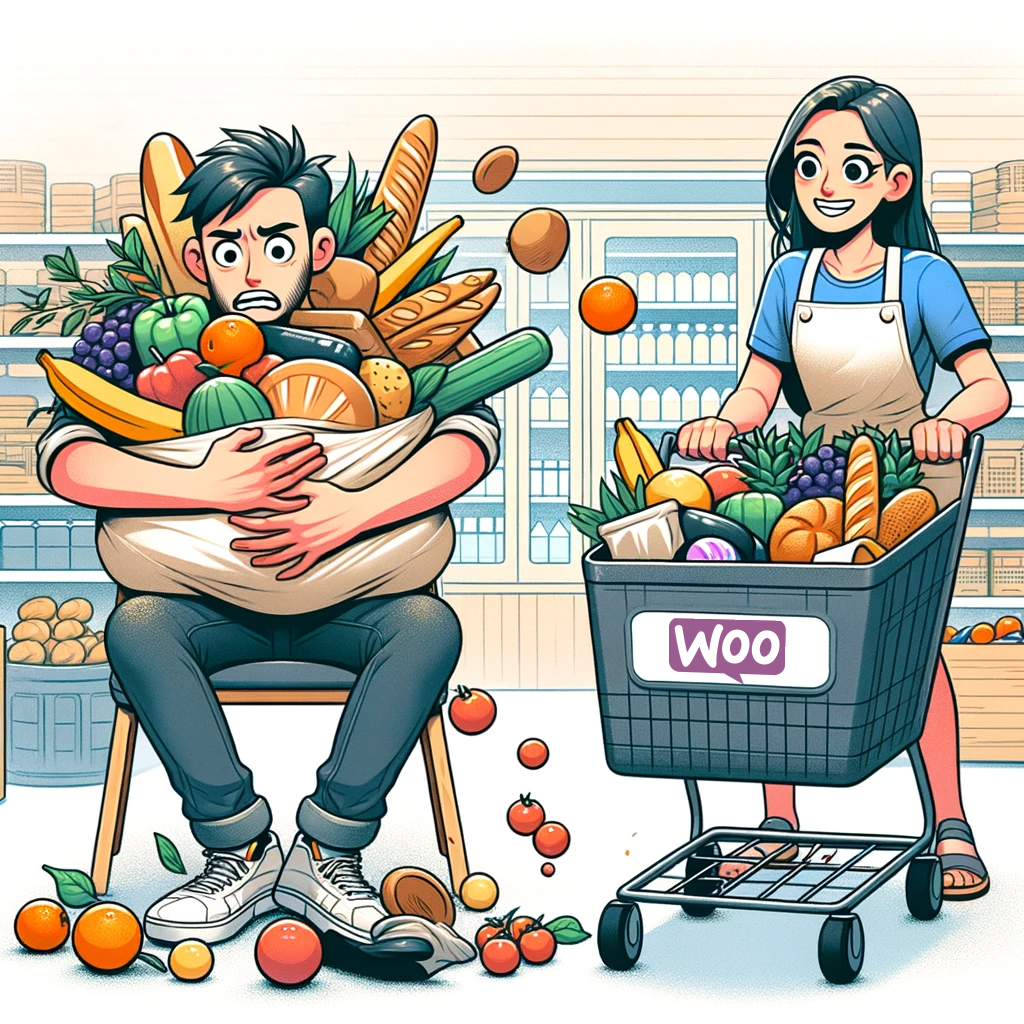
Why Should You Customize Your WooCommerce API Responses?
Customizing your WooCommerce API responses can have a profound impact on how you manage, operate, and grow your e-commerce business. The API offers specialized endpoints for handling WooCommerce-specific data such as products, orders, customers, and coupons. This flexibility is crucial for several reasons:
- Interoperability Across Platforms: One of the key benefits of using the WooCommerce REST API is its ability to seamlessly integrate with various platforms and applications. This interoperability is vital for e-commerce stores that operate across different digital ecosystems, ensuring consistent data management and user experiences across all platforms.
- Customized User Experiences: The ability to customize API responses means you can tailor the data and functionality of your WooCommerce store to meet the specific needs of your customers. This can range from personalized product recommendations to custom checkout processes, significantly enhancing the overall user experience.
- Automating and Streamlining Operations: With customized API responses, you can automate many aspects of your e-commerce operations. For instance, you can integrate with inventory management systems to keep stock levels updated in real-time, or connect with CRM systems to synchronize customer data automatically.
- Creating Custom Mobile Apps: As mobile commerce continues to grow, having a custom mobile app for your store becomes increasingly important. The WooCommerce REST API allows you to develop such apps, leveraging the API to manage products, process orders, and handle customer authentication.
- Advanced Product Search and Filtering: For larger stores, offering advanced search and filtering options becomes necessary to improve customer experience. The API allows for the creation of sophisticated search algorithms that can filter products based on various attributes, categories, or custom criteria.
- Integration with Fulfillment Services: The WooCommerce REST API can be used to integrate with fulfillment services or shipping carriers. This integration can automate the generation of shipping labels and synchronize shipping information, streamlining the shipping process for both the store owner and the customers.
- Developing Custom Pricing or Discount Systems: You can use the API to implement complex pricing models or discount systems, offering a more personalized shopping experience and helping to increase sales and customer loyalty.
- Building Custom Reports and Analytics: The API can be leveraged to build custom reporting features that provide insights into sales patterns, customer behavior, and inventory.
For more information on WooCommerce API endpoints, check out Neil Matthews’ Guide to WooCommerce REST API Endpoints and Creating Custom Endpoints with the WordPress REST API!
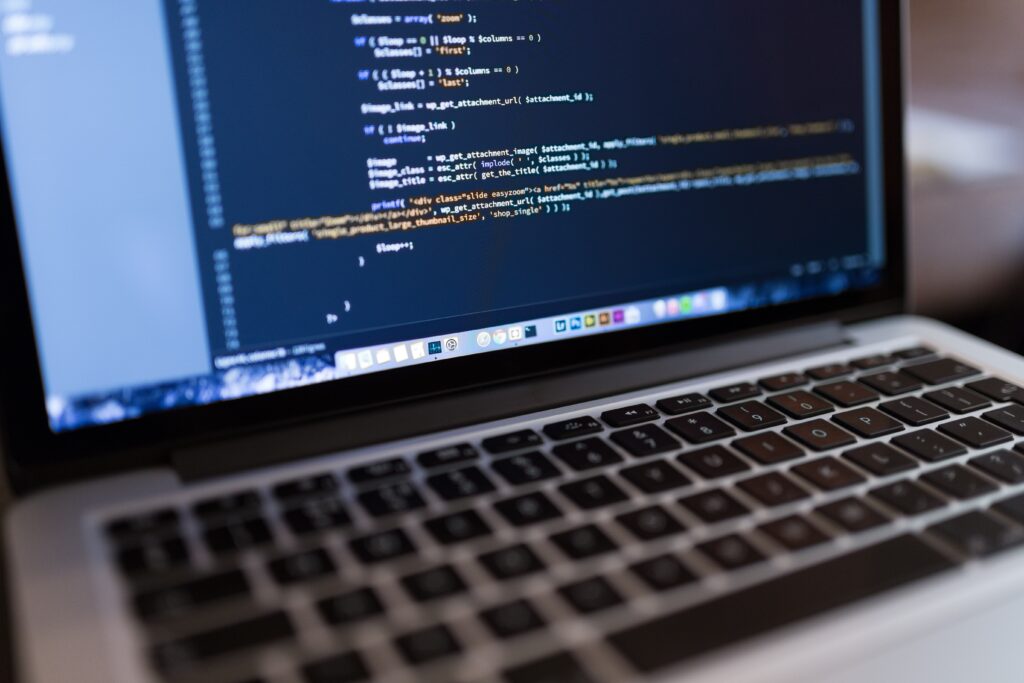
Practical Guide To Customizing Your WooCommerce API Responses
Hooks and Filters in WooCommerce
In WooCommerce, hooks and filters are powerful tools that allow you to modify API responses. Hooks are used to execute an action at a specific point in the execution process, while filters allow you to modify data before it is sent back in an API response.
Modifying Product Responses
Let’s start with customizing product responses. WooCommerce provides a filter named woocommerce_rest_prepare_product_object
. This filter can be used to modify the product data before it’s returned in the API response.
add_filter('woocommerce_rest_prepare_product_object', 'customize_product_response', 10, 3);
function customize_product_response($response, $post, $request) {
// Add custom data to the response
$response->data['custom_field'] = 'Custom Value';
return $response;
}
In this example, we add a new field custom_field
to the product response.
Customizing Order Responses
To customize order responses, you can use the woocommerce_rest_prepare_shop_order_object
filter.
add_filter('woocommerce_rest_prepare_shop_order_object', 'customize_order_response', 10, 3);
function customize_order_response($response, $order, $request) {
// Modify order response data
$response->data['order_custom_field'] = get_post_meta($order->get_id(), '_order_custom_field', true);
return $response;
}
This snippet adds a custom field order_custom_field
to the order response, which is retrieved from the order meta.
Altering Customer Responses
For customizing customer responses, the woocommerce_rest_prepare_customer
filter is useful.
add_filter('woocommerce_rest_prepare_customer', 'customize_customer_response', 10, 3);
function customize_customer_response($response, $customer, $request) {
// Add custom data to customer response
$response->data['customer_preference'] = 'Your Custom Data';
return $response;
}
Here, we’re adding a customer_preference
field to the customer API response.
Advanced Customization: Creating Custom Endpoints
If you need more control, you can create custom endpoints. This allows for more complex modifications and can be used to add entirely new functionalities to your WooCommerce API.
add_action('rest_api_init', function () {
register_rest_route('wc/v3', '/custom-endpoint', array(
'methods' => 'GET',
'callback' => 'custom_endpoint_handler',
));
});
function custom_endpoint_handler($request) {
// Handle the custom endpoint logic
return new WP_REST_Response('Custom Endpoint Data', 200);
}
This code creates a new custom endpoint under the WooCommerce API namespace. When accessed, it returns a custom response. This approach is particularly useful for adding entirely new functionalities or data structures that the default API doesn’t support.
Real-Life Examples
- Adding a Login Requirement for Purchases: You can use action and filter hooks to prompt customers to log in before they can make a purchase, especially for exclusive products.
- Displaying Credit Card Logos: Implement an action hook to display trusted credit card logos on the checkout page, enhancing the legitimacy of your store and informing customers about accepted payment methods.
- Social Media Share Icons: Add share buttons on product pages using action hooks, facilitating easy sharing of your products on social media platforms.
Best Practices and Considerations
- Always test your customizations in a staging environment before applying them to your live site.
- Keep in mind the performance implications of your customizations, especially if you’re adding complex queries or operations.
- Ensure that your customizations are secure and do not expose sensitive data unintentionally.
- Stay updated with WooCommerce and WordPress updates to ensure compatibility.
Customizing your WooCommerce API responses by using hooks and filters is a powerful way to tailor your online store’s functionality to your specific needs. Whether you’re adding simple custom fields, or creating entirely new endpoints, the flexibility offered by the WooCommerce API is a robust tool in the hands of a skilled developer. Remember to apply these customizations thoughtfully, keeping in mind the best practices for a secure and efficient online store.
Additional Resources:
- Customize / Modify WooCommerce REST API response using filter
- WooCommerce Hooks: A Comprehensive Guide
- How to Customize Your Store Using WooCommerce Hooks

Advanced WooCommerce API Customization Strategies
Advanced customization of the WooCommerce API opens up a myriad of possibilities, allowing experienced developers to significantly enhance the efficiency and flexibility of their WooCommerce stores. Here’s a dive into some sophisticated strategies:
1. Leveraging WC_Data for Customizations
WC_Data is a foundational class in WooCommerce, central to most persistent data interactions. This class offers database-agnostic property management, CRUD (Create, Read, Update, Delete) operations, and metadata management. By understanding and utilizing WC_Data
, you can effectively customize various aspects of WooCommerce, from product data to order information.
2. Creating and Loading Custom Data Stores
Custom data stores are a powerful way to abstract database interactions, used extensively by WC_Data
and its child classes. By creating custom data stores, you can tailor how WooCommerce interacts with the database, optimizing performance and adding unique functionalities specific to your store’s needs.
3. Building Custom Interactions with WC_Session, WC_Cart, and WC_Checkout
The combination of WC_Session
, WC_Cart
, and WC_Checkout
allows for the creation of custom shopping experiences. For instance, you could develop a shared cart functionality for users or enhance the checkout process with additional steps or validations. These classes offer extensive hooks and filters for customization.
4. Integrating with External Systems Using REST API and Webhooks
The REST API and Webhooks provide significant opportunities for integrating WooCommerce with external systems, such as inventory management or mobile apps. While the REST API handles data requests to WooCommerce, Webhooks can be configured to push data from WooCommerce to external applications automatically, opening up possibilities for real-time data synchronization and automated workflows.
5. Gutenberg Development for WooCommerce
With the integration of the Gutenberg editor in WordPress, you can build custom WooCommerce Gutenberg blocks. This approach allows you to create unique product displays, customized checkout processes, and other interactive elements that enhance the user experience on your WooCommerce site.
6. Utilizing Custom Action and Filter Hooks
WooCommerce is filled with action and filter hooks that allow for modifications and extensions. By creating custom hooks or utilizing existing ones, you can add or modify functionalities, adjust how data is displayed, or integrate with third-party services.
With the right approach and expertise, these strategies can help create a WooCommerce store that stands out in the competitive e-commerce landscape, by offering a more efficient, personalized, and feature-rich shopping experience for your customers.
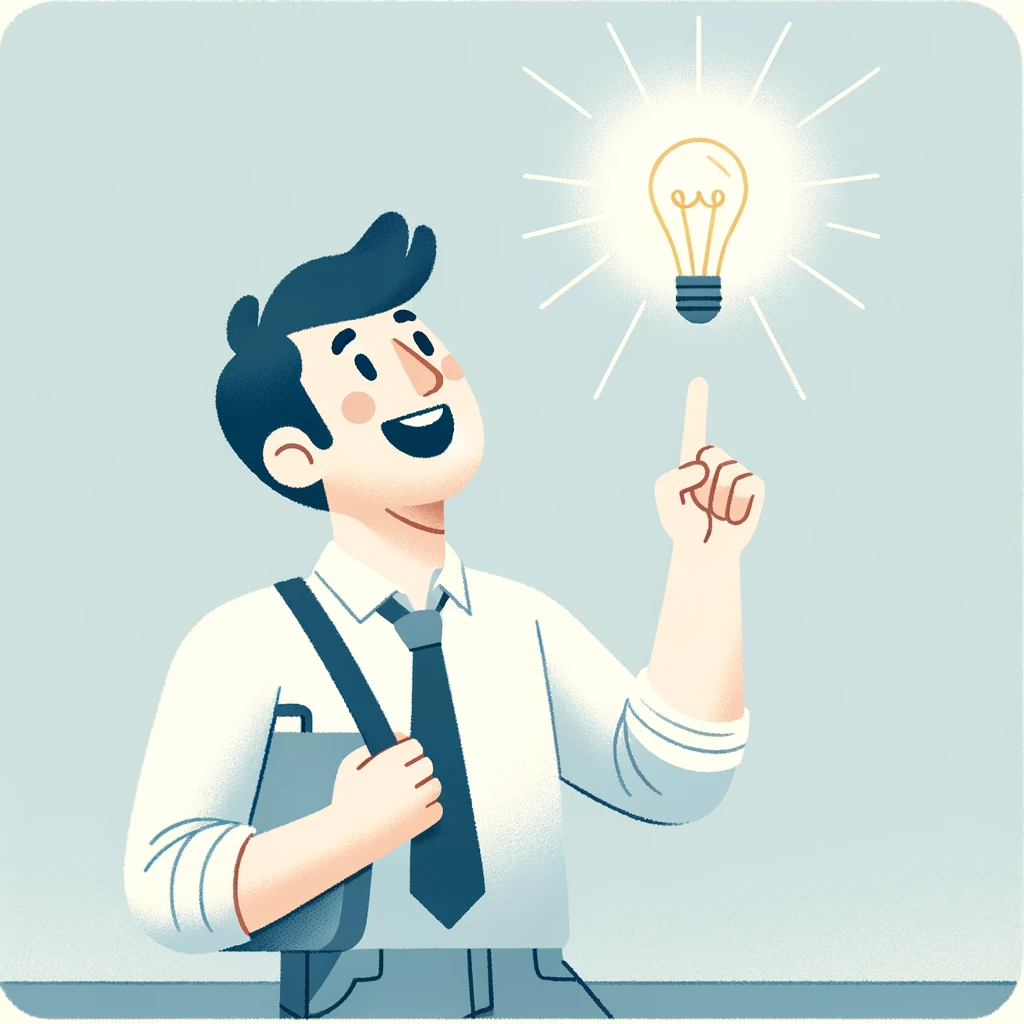
Best Practices & Common Pitfalls in Customizing the WooCommerce API
Customizing WooCommerce API responses can significantly enhance the functionality and user experience of your online store. However, it’s crucial to approach these customizations with best practices in mind to ensure they are efficient, secure, and maintainable. Let’s talk about some key best practices and common pitfalls to avoid when working with the WooCommerce REST API.
Best Practices for Customizing API Responses
- Start with a Clear Objective: Before diving into customizations, have a clear understanding of what you want to achieve. This clarity will guide your approach and help you stay focused on relevant modifications.
- Use Child Themes for Customizations: When adding custom code to your WordPress site, always use a child theme. This practice prevents your customizations from being overwritten when the parent theme is updated.
- Adhere to WordPress Coding Standards: Follow the WordPress coding standards to maintain code quality and consistency, which is especially important for long-term maintenance and team collaboration.
- Implement Proper Authentication and Permissions: Ensure that any custom endpoints or modifications to existing ones have proper authentication and permission checks. This step is vital for maintaining the security of your site’s data.
- Optimize for Performance: Be mindful of the performance impact of your customizations. Avoid overly complex queries and consider caching strategies to minimize the load on your server.
- Regularly Update and Test: Keep your WordPress, WooCommerce, plugins, and themes updated. Regularly test your customizations to ensure compatibility with new versions.
Common Pitfalls and How to Avoid Them
- Modifying Core Files: Avoid modifying any core WooCommerce or WordPress files. These changes can be overwritten with updates and can lead to maintenance headaches. Use hooks and filters instead.
- Ignoring Data Validation and Sanitization: Always validate and sanitize data when modifying API responses. This approach is crucial for preventing security vulnerabilities like SQL injections and cross-site scripting (XSS).
- Overlooking Error Handling: Implement robust error handling in your custom code. Proper error handling can help you debug issues faster and improve the overall reliability of your API.
- Neglecting Mobile Responsiveness: In today’s mobile-first world, ensure that any customizations are responsive and function well across all devices.
- Creating Unnecessary Endpoints: Only create custom endpoints if absolutely necessary. Often, existing endpoints can be modified to meet your needs, which is usually a more efficient approach.
- Forgetting to Backup: Always backup your site before making any changes. This safety net allows you to restore your site in case something goes wrong.
By following these best practices and avoiding common pitfalls, you can customize your WooCommerce API responses in a way that is efficient, secure, and enhances the functionality of your online store. Remember, the goal is to improve your e-commerce platform while maintaining a high standard of coding and security practices. Stay informed about the latest trends and updates in WooCommerce and WordPress development to ensure your customizations remain effective and relevant.

Final Thoughts
We’ve explored various aspects of customizing WooCommerce API responses. From understanding the basic structure to advanced customization strategies, we’ve covered key techniques and best practices to enhance your store’s efficiency, user experience, and functionality while maintaining secure and sustainable practices.
For further exploration and learning, the WooCommerce REST API Documentation is an invaluable resource. It provides detailed insights into API functionalities and customization options. Additionally, for those looking to expand their knowledge in WordPress and WooCommerce development, websites like WordPress Codex and WPBeginner offer a wealth of information.
Your experiences and insights are valuable! Feel free to share your own customization journeys or ask any questions in the comments section below. Your input enriches our community’s knowledge and helps others in their WooCommerce endeavors.
Special Offer from WordPress Whispers
As part of my commitment to empowering your WordPress journey, I invite you to subscribe below. By subscribing, you’ll receive our exclusive eBook on Headless WordPress absolutely free! Plus, gain access to our private LinkedIn group, exclusive content, and early bird access to future courses. This is your chance to take your WordPress projects to new heights with WordPress Whispers!
