Table of Contents
Cross-Origin Resource Sharing (CORS) is a security feature that restricts web applications from making requests to a domain different from the one that served the web application. However, this security measure can sometimes become a hurdle for developers, especially when integrating APIs from different origins. For WordPress developers, understanding CORS is critical when working with the REST API and JavaScript to ensure seamless data exchange and enhance the functionality of websites.
Why Is CORS Pivotal in WordPress and JavaScript Development?
In the context of WordPress, which powers a significant portion of the web, the REST API has become a cornerstone for creating dynamic web experiences. JavaScript, on the other hand, is the lingua franca of the web, enabling interactive and real-time user interfaces. When these two powerful tools come together, they can create highly interactive and dynamic WordPress sites. However, CORS policies can impede this integration, leading to errors and frustration.
This article aims to demystify CORS within the WordPress ecosystem. I’ll walk you through a step-by-step guide on identifying and resolving CORS issues when using the WordPress REST API with JavaScript. By the end of this tutorial, you will have a clear understanding of:
- What CORS is and its implications on web development.
- The importance of handling CORS correctly in WordPress and JavaScript projects.
- Practical steps to implement CORS solutions in a WordPress environment.
Valuable Resources
I’ve scoured the web for the latest insights on CORS and WordPress REST API integration. One valuable resource I found is a discussion on the WordPress Development Stack Exchange, where developers share their experiences and solutions for enabling CORS in WordPress.
Moreover, the Amazon Simple Storage Service (S3) documentation provides a comprehensive overview of CORS and its use-case scenarios, which can be insightful for understanding the concept in a broader context.
Finally, be sure to check out this WordPress Whispers article, A Deep Dive Into WordPress REST API Endpoints, for a thorough explanation of both default and custom REST API endpoints.
Staying Informed
As we embark on this journey together, remember that the path to mastering web development is a marathon, not a sprint. I invite you to follow along with this tutorial, ask questions, and share your experiences in the comments and on social media! I am always happy to personally answer any questions and provide additional resources. Whether you’re a seasoned developer or just starting, WordPress Whispers is here to guide you through the process of overcoming challenges in your WordPress projects.
Let’s dive in and turn those CORS errors into a thing of the past!
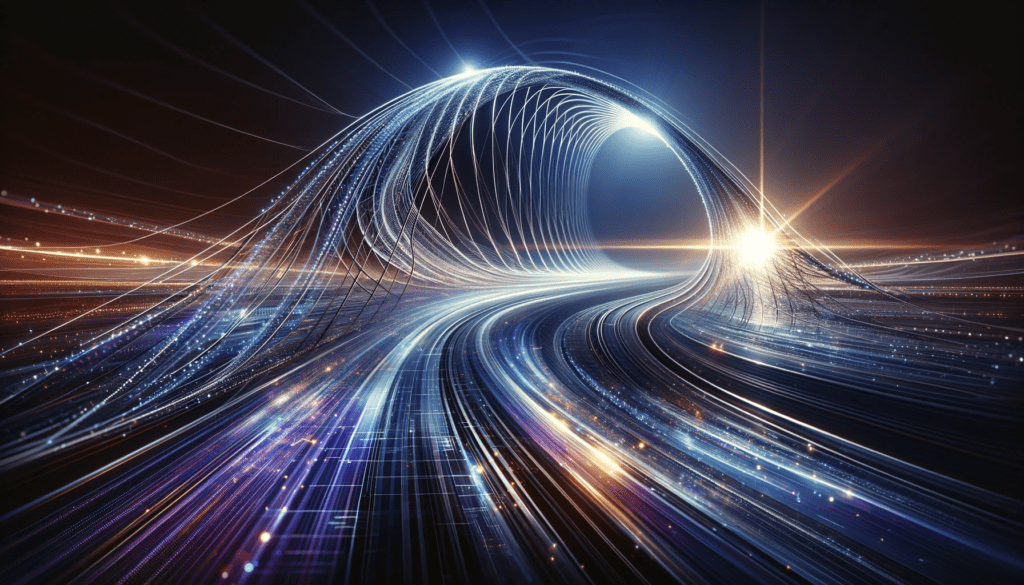
Understanding CORS and the WordPress REST API
Cross-Origin Resource Sharing (CORS) is a crucial web technology that ensures web applications running in one domain can securely access resources from another domain. This mechanism is vital for modern web development, where resources are often distributed across various locations.
How CORS Works with Web Browsers and Servers
CORS operates by allowing servers to specify who can access their assets and under what conditions. When a web application makes a request to a server from a different origin, the browser sends an HTTP request with an Origin
header. The server then decides whether to respond with an Access-Control-Allow-Origin
header, which either grants or denies permission to fetch the resource. If the server’s response includes the requesting origin in the Access-Control-Allow-Origin
header, the browser permits the request to proceed.
The Role of the WordPress REST API in a JavaScript Application
The WordPress REST API plays a pivotal role in decoupling the WordPress content management system from the front-end presentation layer. This separation allows developers to use JavaScript frameworks like React or Vue.js to build dynamic, app-like experiences. The REST API provides a set of routes and endpoints that enable developers to interact with WordPress content—such as posts, pages, and custom post types—in a programmatic way using JavaScript.
Common Scenarios Where CORS Issues Arise with WordPress
CORS issues often arise when a WordPress site’s REST API is accessed from a JavaScript application hosted on a different domain. For instance, if you’re developing a headless WordPress site and your front-end application tries to fetch data from the WordPress backend, you may encounter CORS errors if the server is not configured correctly to handle cross-origin requests.
By understanding CORS and configuring it correctly, you can ensure that your JavaScript applications interact seamlessly with the WordPress REST API, regardless of where they are hosted.
Resources:
MDN Web Docs on CORS
Auth0’s CORS Tutorial
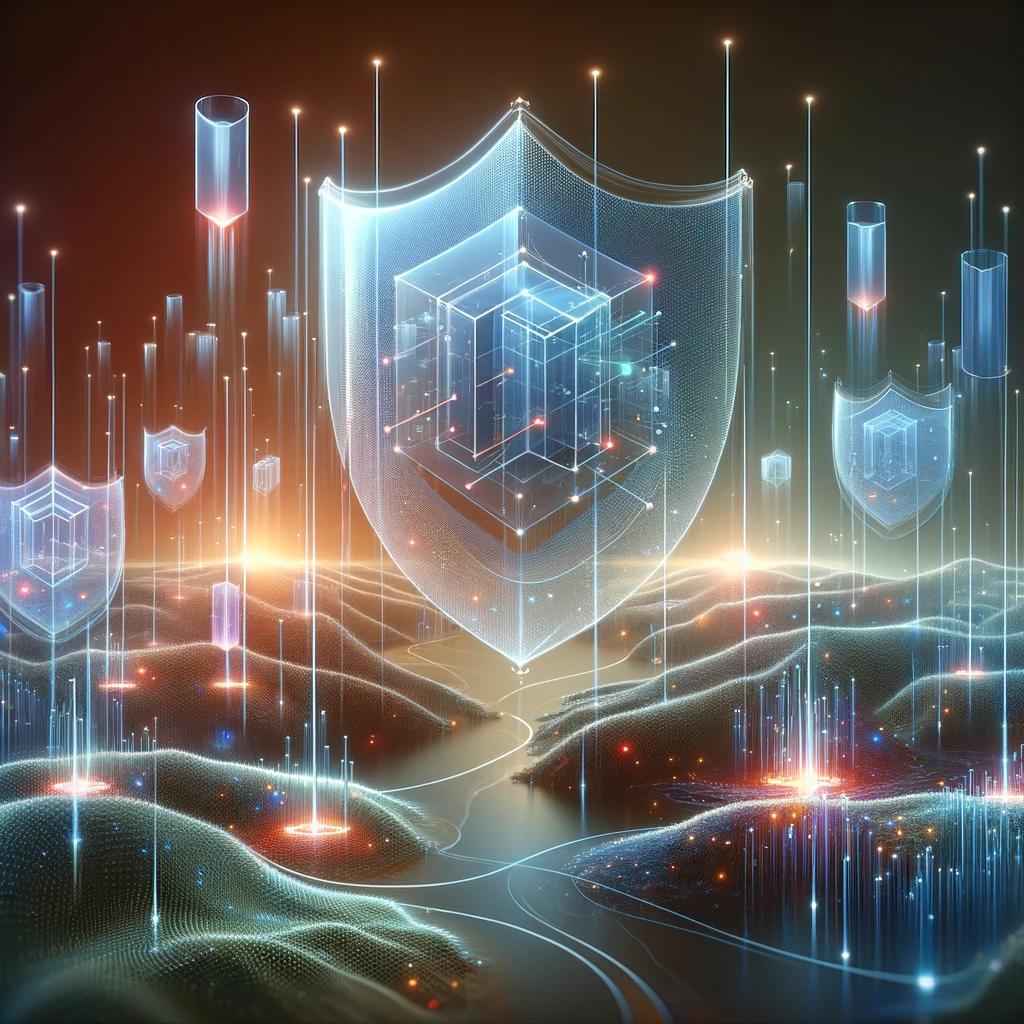
Setting Up Your WordPress Environment for CORS
Setting up your WordPress environment to handle CORS (Cross-Origin Resource Sharing) is a crucial step in ensuring that your website can securely and efficiently interact with other domains. This is particularly important when you are working with JavaScript applications that need to make requests to the WordPress REST API from a different domain.
Here’s how you can configure your WordPress website for CORS compatibility, keeping in mind the latest best practices and security considerations:
Configuring WordPress for CORS Compatibility
1. Modify .htaccess
or web.config
Files: If you’re running an Apache server, you can add the following lines to your .htaccess
file:
Header set Access-Control-Allow-Origin "*"
Header set Access-Control-Allow-Methods "GET, POST, OPTIONS, PUT, DELETE"
Header set Access-Control-Allow-Headers "Content-Type, Authorization"
For Nginx servers, such as those used by Flywheel, you can add the following to your server configuration:
add_header 'Access-Control-Allow-Origin' '*';
add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS, PUT, DELETE';
add_header 'Access-Control-Allow-Headers' 'Content-Type, Authorization';
And for IIS servers, you can modify the web.config
file with similar settings.
2. Use a Plugin: There are plugins available, such as Enable Cors that can help you manage CORS settings with ease. These plugins typically offer a user-friendly interface to specify allowed domains and methods.
3. Code Snippets: You can also insert code snippets into your theme’s functions.php
file to enable CORS. Here’s an example snippet:
function add_cors_http_header(){
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Methods: GET, POST, OPTIONS, PUT, DELETE");
header("Access-Control-Allow-Headers: Content-Type, Authorization");
}
add_action('init','add_cors_http_header');
Remember to replace the asterisk (*) with your specific domain if you want to restrict access to known sites.
Security Considerations
- Specify Domains: It’s recommended to specify which domains are allowed to access your resources rather than using a wildcard (*). This minimizes the risk of unauthorized access.
- Limit Methods: Only allow necessary HTTP methods. If your application doesn’t need to perform actions like PUT or DELETE, don’t include them in the
Access-Control-Allow-Methods
header. - Secure Credentials: Be cautious with credentials and sensitive data. If your requests include authentication, ensure that the
Access-Control-Allow-Origin
header is not set to a wildcard.
Helpful Resources
- WordPress Plugin Directory: Search for CORS-related plugins.
- Stack Overflow: Community Q&A for troubleshooting specific CORS issues on various server setups.
- Mozilla Developer Network: In-depth explanations of CORS and its headers.
By following these steps and considerations, you can set up your WordPress environment to handle CORS effectively. Always test your configuration in a safe environment before deploying changes to your live site to avoid any disruptions.
Remember, while these configurations can help you manage CORS issues, it’s always important to stay updated with the latest security practices to protect your website and its users.
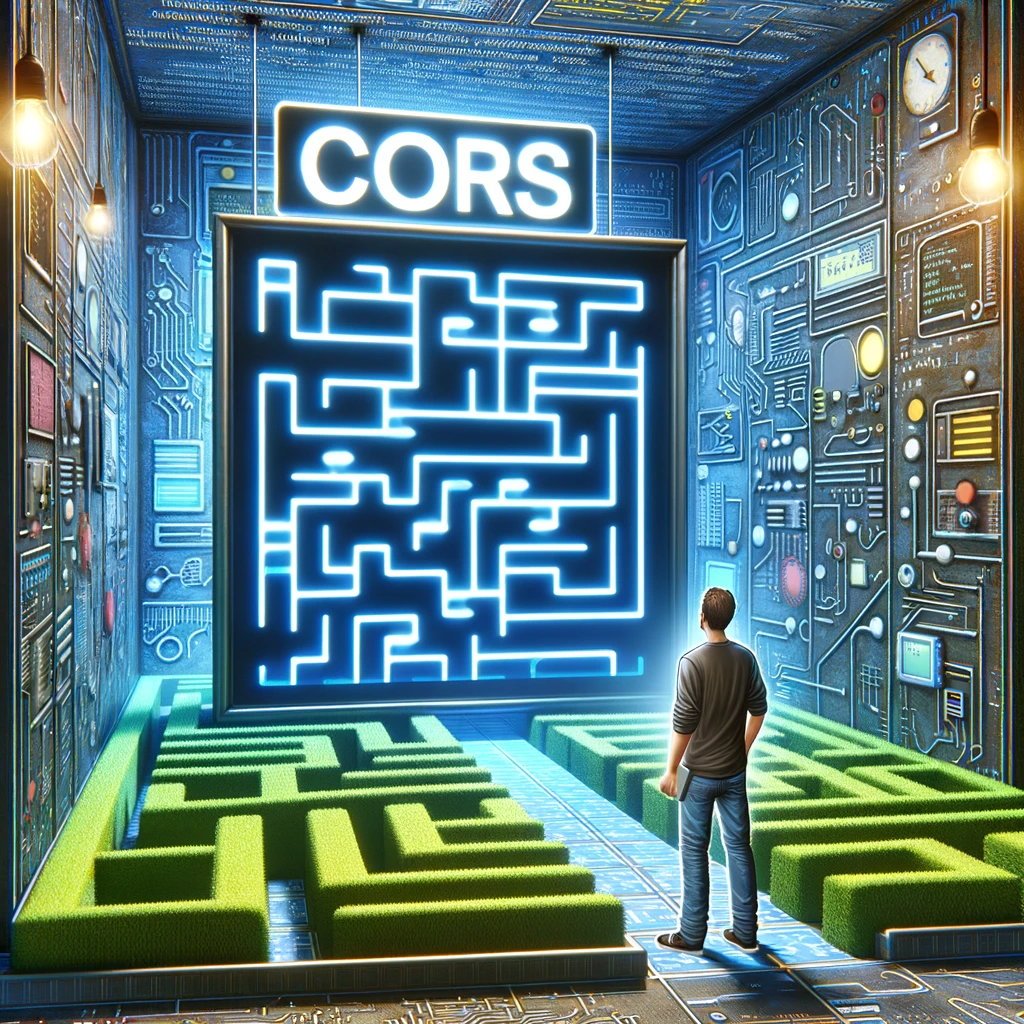
Front-End JavaScript and WordPress REST API Integration
Implementing front-end solutions in JavaScript to handle CORS errors involves a few strategic steps. Here’s a friendly guide to ensure your JavaScript applications communicate smoothly with the WordPress REST API.
Writing JavaScript Code to Handle CORS Errors
When a CORS error occurs, it’s typically because the server you’re requesting resources from doesn’t include the proper CORS headers in the response to your JavaScript application. To handle these errors gracefully, you can write JavaScript code that tries the request and catches any errors.
Here’s a basic example using the fetch
API, which is a modern approach to making HTTP requests in JavaScript:
fetch('https://your-wordpress-site.com/wp-json/')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('There has been a problem with your fetch operation:', error));
Utilizing Modern JavaScript Features
Modern JavaScript provides elegant ways to handle asynchronous operations like HTTP requests. Features like Promises, async/await, and the Fetch API have made it easier to write clean and efficient code.
For instance, using async/await
alongside the fetch
API simplifies the syntax further:
async function fetchData() {
try {
const response = await fetch('https://your-wordpress-site.com/wp-json/');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('There was an error fetching the data', error);
}
}
fetchData();
JavaScript Code Examples for WordPress Context
In a WordPress context, you might be interacting with the REST API to fetch posts, update content, or manage users. Here’s an example of how you might fetch posts from a WordPress site using JavaScript with proper CORS handling:
async function fetchPosts() {
try {
const response = await fetch('https://your-wordpress-site.com/wp-json/wp/v2/posts');
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const posts = await response.json();
console.log(posts);
} catch (error) {
console.error('Fetch error:', error);
}
}
fetchPosts();
Resources and Further Reading
For the latest information on JavaScript features for handling HTTP requests, a comprehensive guide can be found on Kinsta’s blog, which provides a deep dive into the fetch
API, XMLHttpRequest
, and libraries like Axios and SuperAgent.
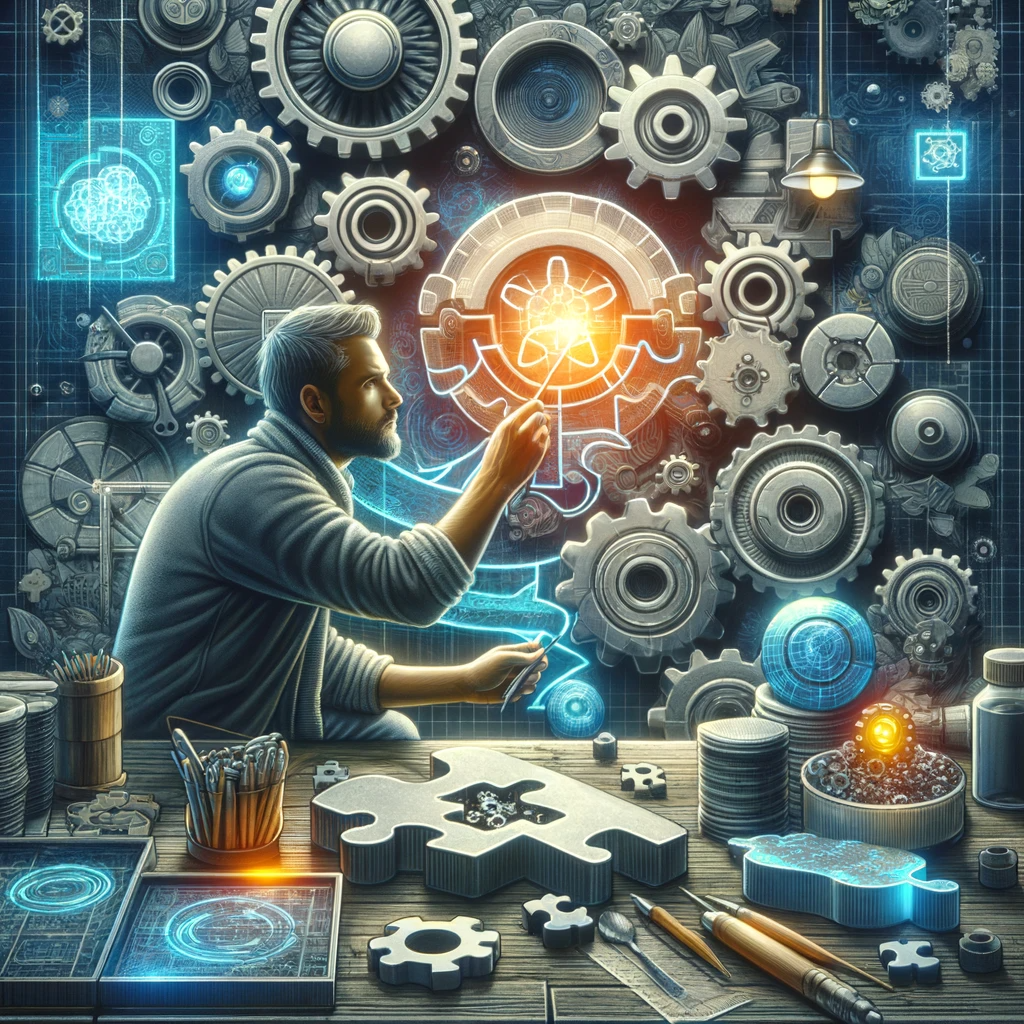
Backend Solutions: PHP and the WordPress REST API
Creating custom REST endpoints in WordPress is a powerful way to extend the capabilities of your website or application. By doing so, you can communicate with virtually any external application or service, making WordPress an even more robust content management system. Here’s how you can achieve this, including handling Cross-Origin Resource Sharing (CORS) and ensuring backend security.
Creating Custom REST Endpoints in WordPress
To create custom REST endpoints, you can use the register_rest_route()
function provided by WordPress. This function allows you to specify your own routes and handle requests using custom callback functions.
Here’s a basic example of how to add a custom endpoint:
add_action( 'rest_api_init', function () {
register_rest_route( 'my_namespace/v1', '/my_endpoint/', array(
'methods' => 'GET',
'callback' => 'my_custom_endpoint_handler',
) );
} );
function my_custom_endpoint_handler( WP_REST_Request $request ) {
// Handle the request and return response
return new WP_REST_Response( array( 'message' => 'This is my awesome custom endpoint!' ), 200 );
}
Handling CORS in WordPress REST API
CORS is a security feature that restricts web applications from making requests to a domain different from the one that served the web application. However, when you’re working with APIs, you might need to allow requests from other origins.
To handle CORS in your custom endpoints, you can send the appropriate headers using PHP. Here’s a snippet that sets the Access-Control-Allow-Origin
header:
add_action( 'rest_api_init', function () {
remove_filter( 'rest_pre_serve_request', 'rest_send_cors_headers' );
add_filter( 'rest_pre_serve_request', function( $value ) {
header( 'Access-Control-Allow-Origin: *' );
header( 'Access-Control-Allow-Methods: POST, GET, OPTIONS, PUT, DELETE' );
header( 'Access-Control-Allow-Credentials: true' );
return $value;
});
}, 15 );
Please Note: The wildcard *
allows all domains. For better security, replace *
with the specific domain(s) you want to allow.
Ensuring Backend Security While Allowing CORS Requests
While enabling CORS is necessary for API communication, it’s crucial to maintain the security of your backend. Here are some tips:
- Validate and Sanitize Data: Always validate and sanitize the input data in your custom endpoints to prevent common vulnerabilities like SQL injection and XSS attacks.
- Authentication: Implement authentication mechanisms to ensure that only authorized users can access your endpoints. WordPress provides several methods for API authentication, such as cookie authentication, application passwords, OAUTH, and JSON Web Tokens.
- Permission Checks: Use permission callbacks in your route registration to check user capabilities before processing the request.
Helpful Resources
For the latest information and best practices on creating custom REST endpoints in WordPress, the following resources can be helpful:
By following these guidelines and utilizing the resources provided, you can effectively create custom REST endpoints in WordPress, handle CORS, and maintain a secure backend.
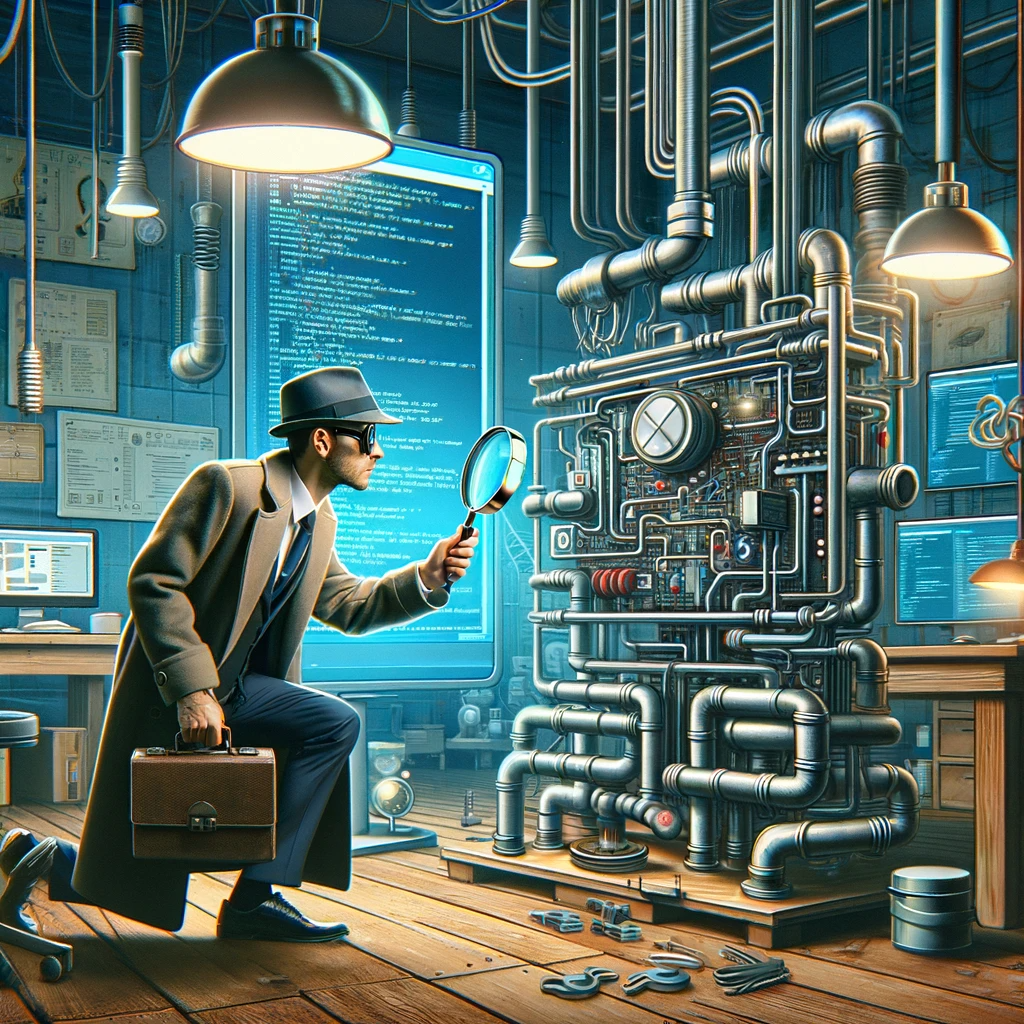
Testing and Debugging
Testing and debugging are critical steps in ensuring that your WordPress site is properly handling CORS issues. Here’s a step-by-step guide to help you through the process, along with some debugging techniques and tools that can assist you.
Step-by-Step Guide to Testing Your WordPress Site for CORS Issues
- Use Browser Developer Tools: Open the developer tools in your browser and go to the ‘Network’ tab. This will allow you to see all the HTTP requests made by your page and their responses.
- Check for Errors: Look for any requests that have failed due to CORS errors. These will typically be marked with a different color or icon and will have an associated error message.
- Inspect HTTP Headers: Select a request that has failed and inspect the HTTP headers. Ensure that the
Access-Control-Allow-Origin
header is present and correctly configured.
Debugging Techniques for JavaScript and PHP Code
- JavaScript Console Logging: Use
console.log()
to output the response from your fetch or XMLHttpRequest calls to see what’s being returned by the server. - PHP Error Logging: Ensure that your PHP error logging is enabled. You can then check the error logs to see if there are any issues with the server-side code.
Tools and Plugins to Assist in Resolving CORS Errors
- CORS Plugin: Install a CORS plugin from the WordPress plugin directory that can help you set the necessary headers without touching code.
- Postman: Use Postman to manually send requests to your WordPress REST API endpoints and analyze the responses.
- REST API Log: A plugin like REST API Log can help you log all REST API requests and responses for easier debugging.
By following these steps and utilizing the tools mentioned, you should be able to test and debug CORS issues on your WordPress site effectively.
Helpful Resources
- WordPress Plugin Directory: Search for CORS-related plugins and tools.
- Browser Developer Tools Documentation: Learn more about using developer tools for debugging.
Remember, the key to successful debugging is to be methodical and patient. Test one change at a time and keep a log of what you’ve tried. This will help you track down the source of the issue more efficiently.
Best Practices and Troubleshooting
When managing CORS in WordPress projects, it’s essential to adhere to best practices to ensure smooth operation and security. Here are some best practices and troubleshooting tips:
Best Practices for Managing CORS in WordPress Projects
- Be Specific with Allowed Origins: Instead of using a wildcard, specify the exact domains that should be allowed to access your resources.
- Use a Consistent Approach: Whether through a plugin or custom code, ensure that your CORS settings are consistent across your WordPress site.
- Keep WordPress Updated: Regular updates to WordPress and plugins can resolve underlying issues that may affect CORS.
- Leverage Hooks and Filters: Use WordPress hooks and filters to modify CORS headers when necessary.
Troubleshooting Common Problems and How to Solve Them
- Missing Headers: If CORS headers are missing, ensure that your .htaccess file or WordPress hooks for headers are correctly configured.
- Cache Issues: Sometimes, caching plugins or server-side caching can serve outdated headers. Clear caches after making changes to CORS settings.
- Plugin Conflicts: Deactivate plugins one by one to identify if a plugin is causing the CORS issue.
Remember, CORS issues can be tricky, but with the right approach, they are solvable. Keep your changes documented, and don’t hesitate to reach out to the community for support.

Final Thoughts
Throughout this article, we’ve navigated the complexities of Cross-Origin Resource Sharing (CORS) and its implications for your WordPress site. We began with a foundational understanding of CORS and why it’s a pivotal aspect of modern web development, especially when interfacing with the WordPress REST API using JavaScript.
We then moved on to practical solutions, detailing how to identify CORS errors, set up your WordPress environment to prevent such issues, and implement front-end solutions in JavaScript. We also discussed backend solutions involving PHP and the importance of testing and debugging to ensure a seamless user experience.
Share Your Experiences!
Now, it’s your turn to take these insights and apply them to your WordPress projects! I invite you to share your success stories or any challenges you encountered along the way. Your feedback is invaluable as it helps us all grow and learn together!
If you’ve found this guide helpful, I encourage you to comment below with your experiences. Should you need further assistance with CORS issues, or if you’re looking for a consultation to delve deeper into this topic, don’t hesitate to reach out!
Remember, the world of web development is constantly evolving, and staying informed about best practices is key to success. For the latest information and resources on CORS and WordPress, keep an eye on our blog and join our community discussions.
I look forward to hearing about how you’ve implemented these solutions and the positive impact they’ve had on your projects. Together, let’s continue to push the boundaries of what’s possible with WordPress and JavaScript.
Stay Connected!
Join our growing community of WordPress enthusiasts! Subscribe today and get exclusive insights, tips, and updates straight to your inbox. Plus, you’ll receive our free eBook on Headless WordPress as a welcome gift. Don’t miss out on the conversation!

Pingback: Unlocking the Power of WordPress PWAs