Table of Contents
Welcome back, WordPress developers and enthusiasts! In the ever-evolving landscape of web development, staying updated with the latest technologies and techniques is crucial. One such technique that has gained significant attention is data fetching in the WordPress REST API.
The WordPress REST API provides an interface for applications to interact with your WordPress site, sending and receiving data as JSON objects. It’s the foundation of the WordPress Block Editor and can enable your theme, plugin, or custom application to present new, powerful interfaces for managing and publishing your site content.
Efficient data fetching is not just about getting the data you need. It’s about getting it quickly and in a way that enhances your website’s performance. When done right, it can significantly improve your website’s load times, user experience, and overall performance.
In this post, we’ll delve into the world of data fetching in the WordPress REST API. We’ll explore how to optimize your data fetching processes for enhanced performance, discuss common pitfalls, and provide solutions to avoid them.
Whether you’re a seasoned WordPress developer or just starting, this post will equip you with the knowledge and skills to master data fetching in the WordPress REST API. So, let’s get started!
Resources:
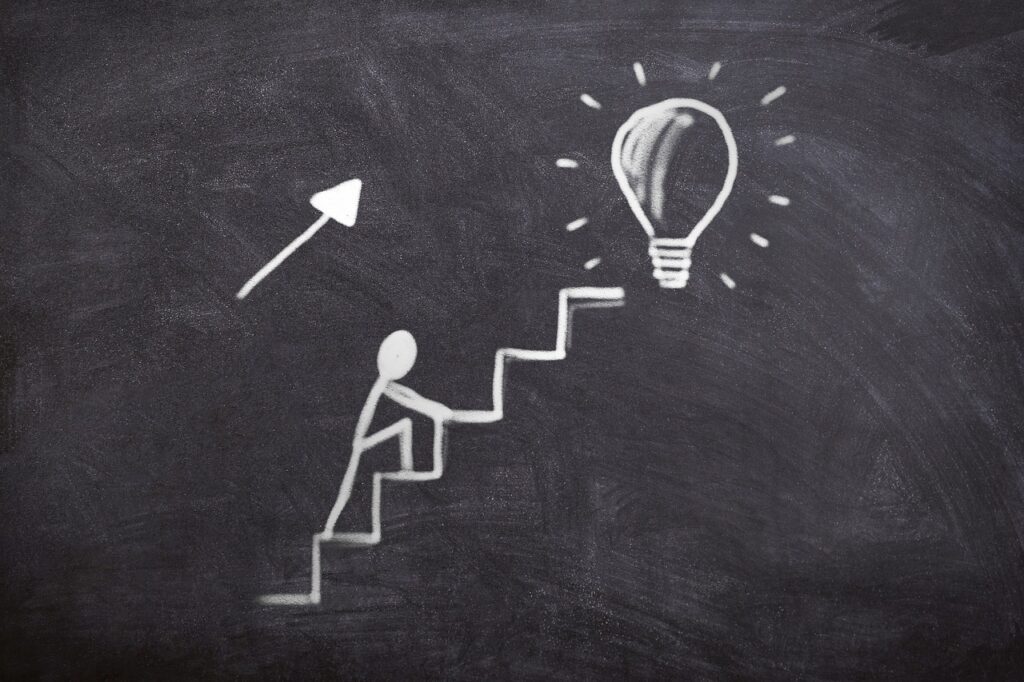
Understanding the WordPress REST API
In the realm of WordPress development, the WordPress REST API has emerged as a powerful tool that allows developers to interact with sites remotely by sending and receiving JSON (JavaScript Object Notation) objects. This API is a key component of the WordPress infrastructure, underpinning the WordPress Block Editor and enabling the creation of more interactive and dynamic interfaces for managing and publishing site content.
The WordPress REST API is built on the concept of REST (REpresentational State Transfer), a software architectural style that defines a set of constraints for creating web services. In the context of the WordPress REST API, RESTful principles allow developers to use HTTP methods to create, read, update, and delete data from your WordPress site.
The WordPress REST API provides various data types and endpoints that developers can interact with. For instance, it includes endpoints for posts, comments, terms, users, meta, and more. Each of these endpoints represents a specific type of data in WordPress. For example, the /wp/v2/posts
endpoint is used to interact with post data.
Here’s a simple example of how to fetch post data using the WordPress REST API:
fetch('https://yourwebsite.com/wp-json/wp/v2/posts')
.then(response => response.json())
.then(posts => console.log(posts));
In this code snippet, we’re using the fetch
function to send a GET request to the posts endpoint of the WordPress REST API. The API responds with a JSON object containing the post data, which we then log to the console.
Mastering the WordPress REST API and its data fetching capabilities is crucial for modern WordPress development. It not only allows you to create more dynamic and interactive websites but also plays a significant role in enhancing your website’s performance.
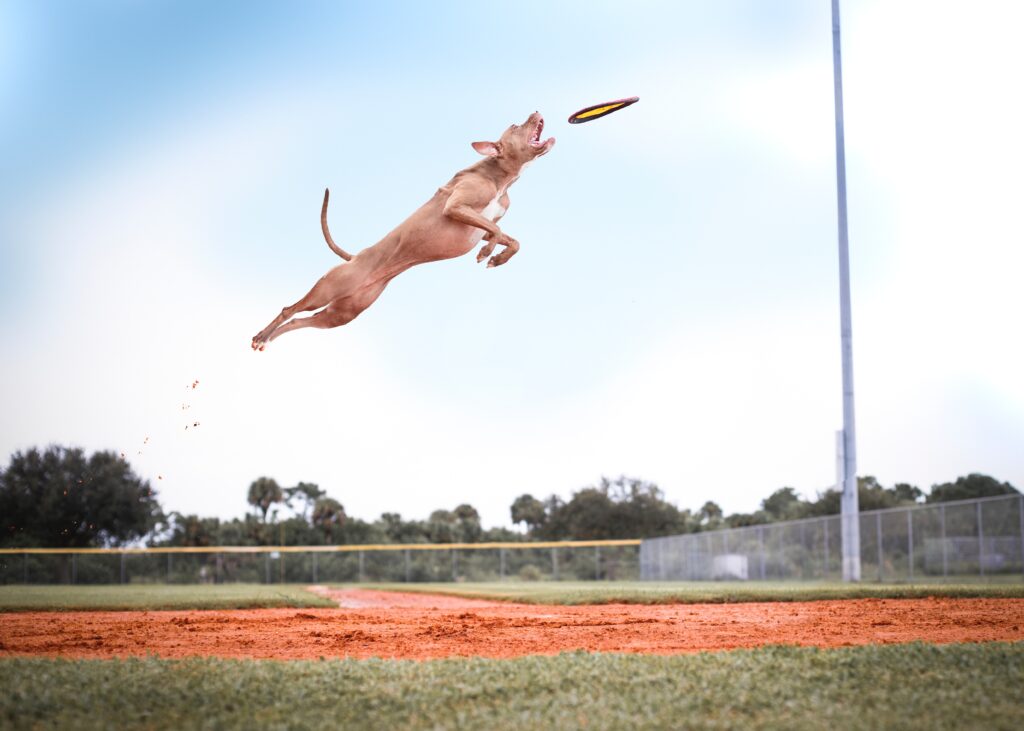
The Importance of Mastering Efficient Data-Fetching
In the world of web development, data fetching is a fundamental task that involves requesting and receiving data from a server or a database. When working with the WordPress REST API, efficient data fetching is not just a nice-to-have, it’s a necessity.
Efficient data fetching can significantly improve your website’s performance. It ensures that your website loads quickly, providing a better user experience and potentially boosting your site’s SEO rankings. On the other hand, poor data fetching practices can lead to performance issues. For instance, fetching more data than necessary can slow down your website, as it takes longer to process and display the data. Similarly, fetching data too frequently can put unnecessary load on your server, leading to slower response times.
Consider this example: let’s say you’re building a blog page that displays a list of posts. Each post includes a title, an excerpt, and a featured image. If you fetch all the data for each post, including the full content, author information, and comments, you’re fetching more data than necessary. This can slow down your website, as it takes longer to process and display the data. Instead, you should only fetch the data you need – in this case, the title, excerpt, and featured image.
fetch('https://yourwebsite.com/wp-json/wp/v2/posts?_fields=title,excerpt,featured_media')
.then(response => response.json())
.then(posts => console.log(posts));
In this code snippet, we’re using the _fields
parameter to limit the data fetched to only the title, excerpt, and featured image. This is an example of efficient data fetching, which can significantly improve your website’s performance.
Mastering data fetching in the WordPress REST API is crucial for optimizing your website’s performance. By understanding how to fetch data efficiently, you can build faster, more responsive websites that provide a better user experience.
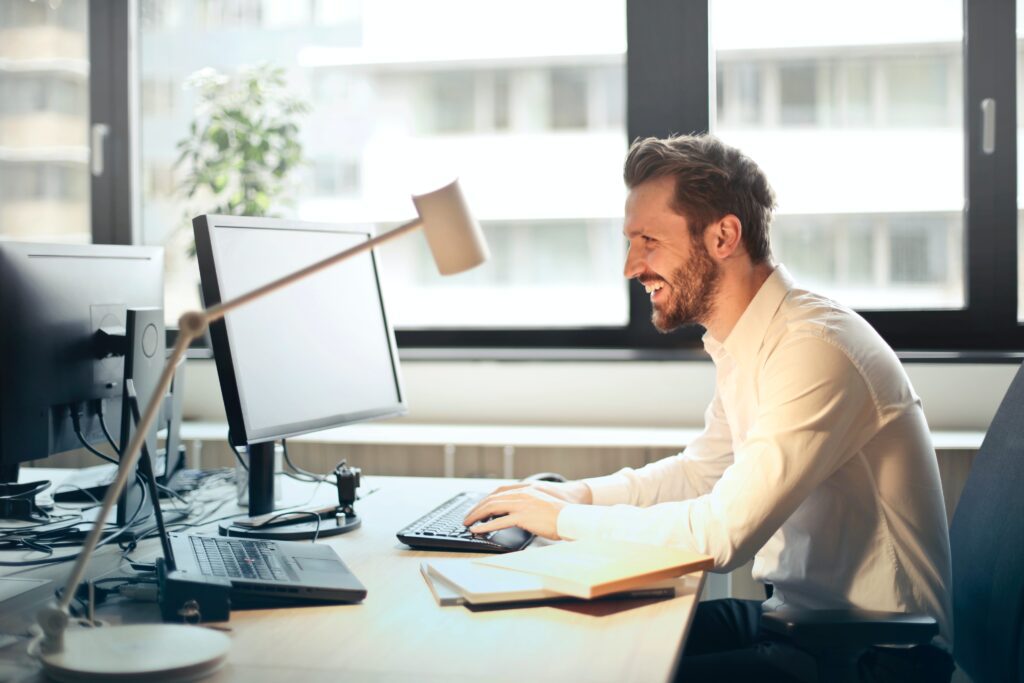
Data Fetching in the WordPress REST API
Data fetching is a fundamental task in web development. It involves requesting and receiving data from a server or a database. In the context of the WordPress REST API, data fetching allows developers to interact with WordPress data remotely, sending and receiving JSON objects.
Fetching data using the WordPress REST API is straightforward. The API provides various endpoints that represent different types of data in WordPress. For instance, you can fetch posts, comments, users, and more using their respective endpoints. The data is sent and received as JSON objects, making it easy to work with in JavaScript.
Here’s an example of how to fetch user data using the WordPress REST API:
fetch('https://yourwebsite.com/wp-json/wp/v2/users')
.then(response => response.json())
.then(users => console.log(users));
In this code snippet, we’re using the fetch
method to send a GET request to the users endpoint of the WordPress REST API. The API responds with a JSON object containing the user data, which we then log to the console.
Understanding how to fetch data using the WordPress REST API is crucial for modern WordPress development. It allows you to create more dynamic and interactive websites and plays a significant role in enhancing your website’s performance.
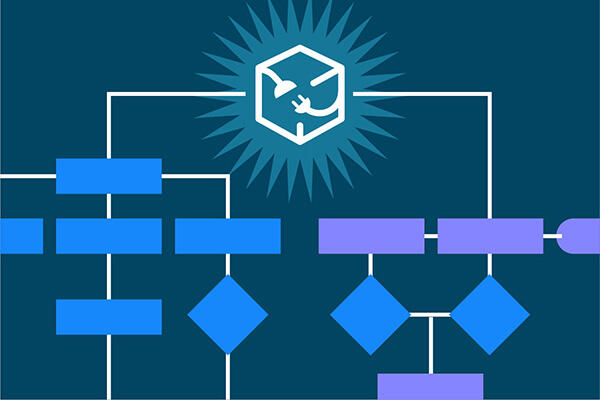
Optimizing Data Fetching for Enhanced Performance
When it comes to data fetching in the WordPress REST API, efficiency is key. Optimizing your data fetching processes can significantly improve your website’s performance, providing a better user experience and potentially boosting your site’s SEO rankings. Here are some strategies you can use to optimize data fetching in the WordPress REST API:
1. Pagination
The WordPress REST API supports pagination out of the box, allowing you to fetch data in smaller, more manageable chunks. This can significantly improve your website’s performance, especially when dealing with large amounts of data. Here’s an example of how to fetch the first 10 posts using pagination:
fetch('https://yourwebsite.com/wp-json/wp/v2/posts?per_page=10')
.then(response => response.json())
.then(posts => console.log(posts));
2. Limiting Fields
By default, the WordPress REST API returns all fields for each resource. However, you can limit the fields returned by the API to only those you need, reducing the amount of data transferred and improving performance. Here’s an example of how to fetch only the id and title fields for posts:
fetch('https://yourwebsite.com/wp-json/wp/v2/posts?_fields=id,title')
.then(response => response.json())
.then(posts => console.log(posts));
3. Caching
Caching involves storing the results of data fetching operations and reusing them for subsequent requests, reducing the load on your server and improving performance. The WordPress REST API supports caching out of the box, and you can further optimize it using various caching plugins or server configurations.
By implementing these strategies, you can optimize your data fetching processes in the WordPress REST API, leading to enhanced performance and a better user experience.
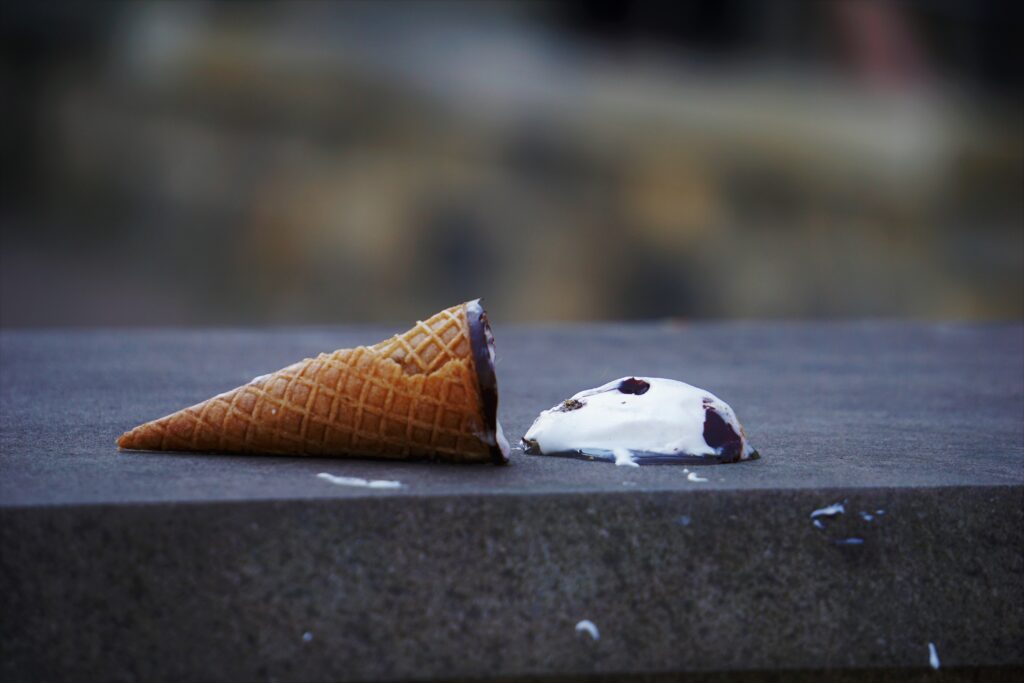
Common Pitfalls when Data Fetching and How To Avoid Them
While the WordPress REST API provides a powerful interface for fetching data, developers can sometimes fall into certain pitfalls that can affect their website’s performance. Here are some common mistakes and how to avoid them:
1. Fetching More Data Than Necessary
One common mistake is fetching more data than necessary. For instance, fetching all fields for a resource when you only need a few can slow down your website and put unnecessary load on your server. To avoid this, use the _fields
parameter to limit the fields returned by the API to only those you need.
fetch('https://yourwebsite.com/wp-json/wp/v2/users?_fields=id,name')
.then(response => response.json())
.then(users => console.log(users));
2. Not Using Pagination
Another common mistake is not using pagination when dealing with large amounts of data. Fetching all data at once can slow down your website and lead to performance issues. To avoid this, use the per_page
parameter to fetch data in smaller, more manageable chunks.
fetch('https://yourwebsite.com/wp-json/wp/v2/posts?per_page=10')
.then(response => response.json())
.then(posts => console.log(posts));
3. Not Utilizing Caching
Not utilizing caching is another common pitfall. Caching involves storing the results of data fetching operations and reusing them for subsequent requests, reducing the load on your server and improving performance. The WordPress REST API supports caching out of the box, and you can further optimize it using various caching plugins or server configurations.
By avoiding these common pitfalls, you can optimize your data fetching processes in the WordPress REST API, leading to enhanced performance and a better user experience.
Final Thoughts
In the ever-changing landscape of WordPress development, mastering data fetching in the WordPress REST API is no longer a luxury, but a necessity. Throughout this post, we’ve explored the significance of efficient data fetching, discussed how to fetch data using the WordPress REST API, and delved into strategies for optimizing data fetching for enhanced performance.
We’ve learned that efficient data fetching can significantly improve your website’s performance, providing a better user experience and potentially boosting your site’s SEO rankings. We’ve also discussed common pitfalls in data fetching, such as fetching more data than necessary, not using pagination, and not utilizing caching, and provided solutions to avoid these pitfalls.
As we’ve seen, the WordPress REST API provides a powerful interface for fetching data, allowing developers to create more dynamic and interactive websites. By mastering data fetching in the WordPress REST API, you can take your WordPress projects to the next level, enhancing your website’s interactivity, flexibility, and performance.
Now, it’s your turn to apply these strategies in your WordPress projects. Remember, the key to mastering data fetching in the WordPress REST API is practice. So, start experimenting with different data fetching techniques, and share your tips and findings in the comments below!
Pingback: Mastering CORS Integration in WordPress | WordPress Whispers
Pingback: Efficient Product Management with the WooCommerce API
Pingback: Setting Up a Headless WordPress Environment with React