Table of Contents
Greetings, WordPress heroes! Welcome back to our series of WordPress JavaScript tutorials. Today, we’re diving into an exciting topic that combines the power of JavaScript with the flexibility of WordPress shortcodes. If you’re looking to enhance your WordPress site’s interactivity and functionality, look no further.
WordPress shortcodes are a fantastic feature that allows you to embed files or create objects that would normally require lots of complicated, ugly code, in just one line. They can be used to perform tasks such as embedding files or creating objects and are a staple in the WordPress ecosystem.
The importance of JavaScript for WordPress cannot be overstated. It has become increasingly fundamental to the WordPress admin in recent releases, and is likely to become progressively more important. JavaScript plays a prominent role in WordPress development, and a web developer with adequate knowledge of JS can easily stand out from the crowd by making different modifications and enhancements to a WordPress site.
In this article, we’re going to explore how to enhance WordPress shortcodes with JavaScript. This is a powerful technique that can greatly enhance the functionality and interactivity of your WordPress site. We’ll guide you through the process step by step, ensuring you have a solid understanding by the end of this post. So, whether you’re a seasoned WordPress developer or just getting started, there’s something here for you. Let’s get started!
Understanding WordPress Shortcodes
If you’ve been using WordPress for a while, you’ve probably come across the term “shortcode”. But what exactly are WordPress shortcodes? In a nutshell, a shortcode is a small piece of code, indicated by brackets like [this], that performs a dedicated function on your site. It’s a shortcut that allows you to do nifty things with very little effort.
The importance and benefits of using shortcodes in WordPress are numerous. They simplify the process of adding complex features to your posts or pages. For instance, instead of writing a long, complicated piece of code to embed a video, you can use a simple shortcode. This makes it easier for anyone, even those without coding knowledge, to add interesting functionality to their WordPress site.
Here are a few examples of common shortcodes:
gallery: This shortcode allows you to display a collection of images in a gallery format.
audio: This shortcode lets you embed an audio file.
video: This shortcode enables you to embed a video file.
caption: This shortcode allows you to wrap captions around content.
These are just a few examples. WordPress and many plugins provide a wide range of shortcodes for various functions.
Coming up, we’ll dive deeper into how you can enhance these shortcodes with JavaScript, making them even more powerful and interactive.
Resources:
What Are Shortcodes in WordPress? Explained for Beginners
The Shortcode Block
How to Use WordPress Shortcodes (with Examples)
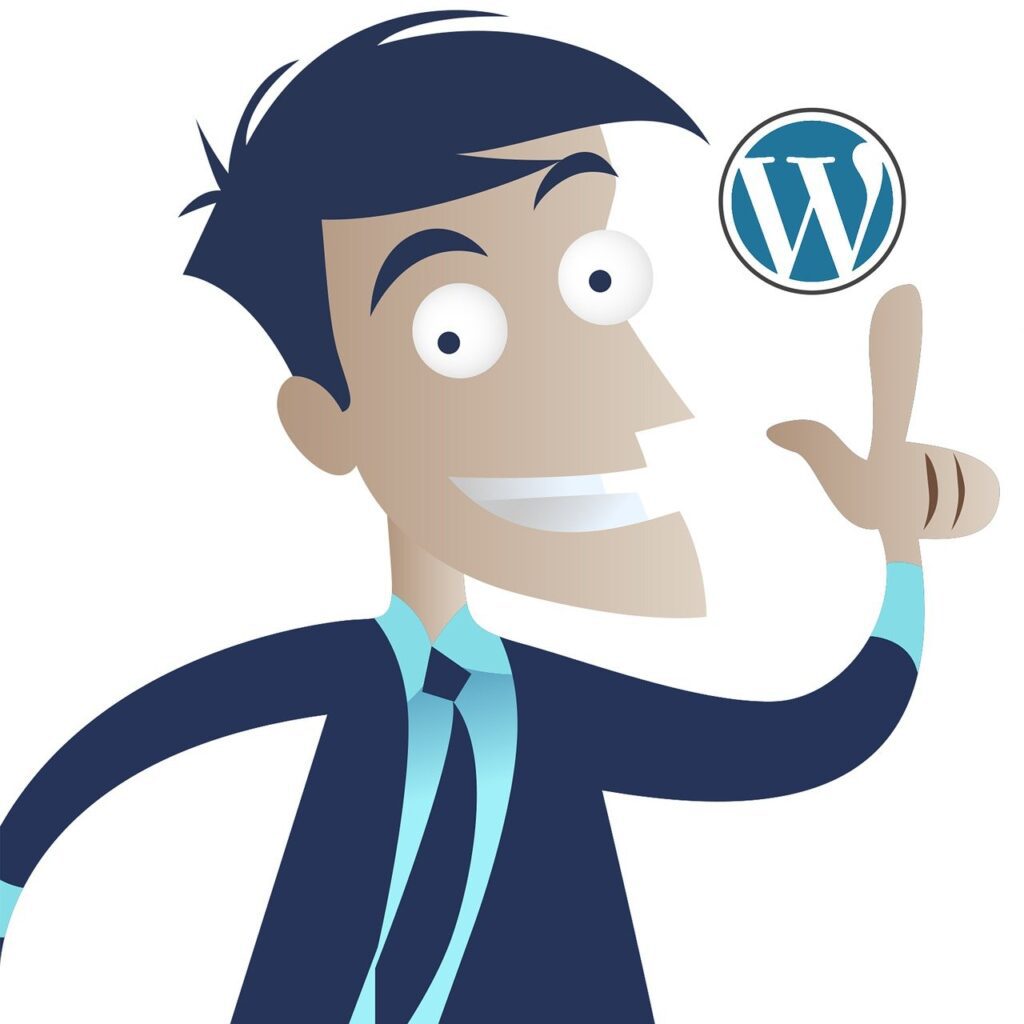
JavaScript and PHP in the Context of WordPress Shortcodes
When it comes to WordPress shortcodes, both JavaScript and PHP play crucial roles. However, they serve different purposes and have unique strengths.
PHP is a server-side scripting language that’s deeply integrated into WordPress. It’s used to create WordPress themes, plugins, and… shortcodes. When you create a shortcode in WordPress, you’re writing a PHP function that WordPress will execute when it encounters your shortcode in a post or page.
JavaScript, on the other hand, is traditionally known as a client-side scripting language, running in the user’s browser. However, with the advent of Node.js, JavaScript can now also be used as a server-side language. This has expanded the possibilities of what JavaScript can do, making it a versatile choice for web development.
So, why would you want to use JavaScript in the context of WordPress shortcodes? Here are a few reasons:
Speed and Performance: JavaScript, particularly when run on the client-side, can update and modify the page without needing to communicate with the server. This can make your shortcodes more responsive and reduce load on your server.
Flexibility and Control: JavaScript is a full-featured programming language, which gives you a lot of power and flexibility. You can use it to create complex, interactive features that would be difficult or impossible to achieve with PHP alone.
Interactivity and User Experience: JavaScript excels at creating interactive features. If you want your shortcode to respond to user input in real time, JavaScript is the tool for the job.
Compatibility with Modern Web Technologies: JavaScript is a core component of many modern web technologies, including AJAX and REST APIs. By using JavaScript in your shortcodes, you can take advantage of these technologies to create more powerful and flexible features.
While PHP is necessary for creating the shortcode itself, JavaScript can greatly enhance what your shortcodes can do. By combining the server-side power of PHP with the versatility of JavaScript, you can create WordPress shortcodes that are truly dynamic and engaging.
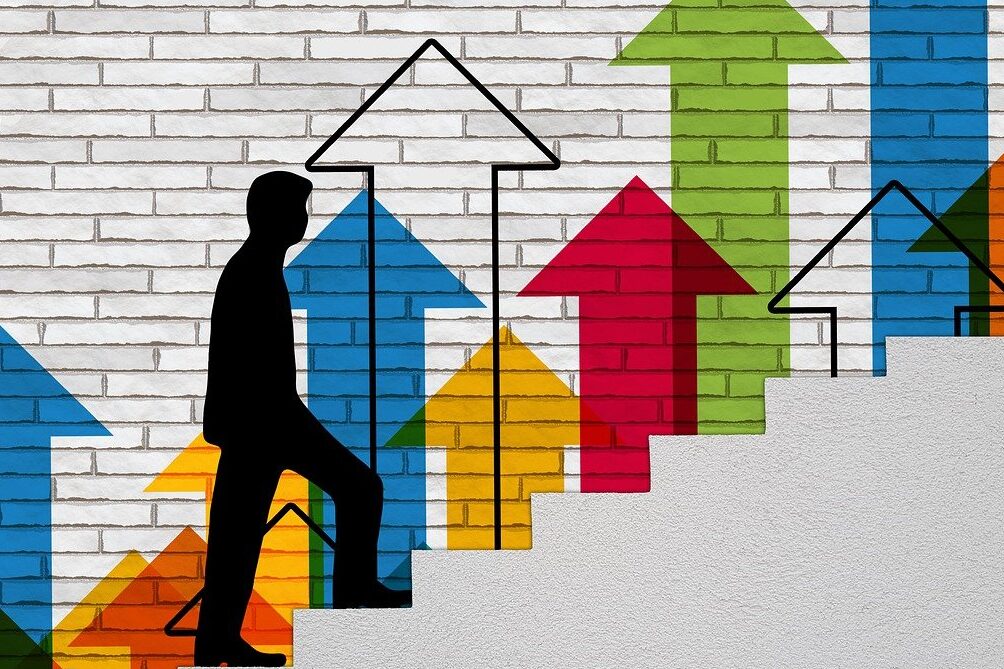
Step-by-step Guide to Enhance WordPress Shortcodes with JavaScript
Let’s jump into the process of enhancing WordPress shortcodes with JavaScript.
Create a JavaScript File
First, create a new JavaScript file in your theme’s directory, such as “custom.js”. This file will contain your JavaScript code. For instance, you can create a function that triggers an alert when a specific element is clicked:
jQuery(document).ready(function($) {
$('#myDiv').click(function() {
alert("Hello, this is my custom JavaScript function!");
});
});
Instantiate Your Shortcode
Next, create a new PHP file in your child theme, such as “custom-shortcode.php”. This file will contain the PHP code that creates your shortcode. Here’s an example of a simple shortcode function:
function my_shortcode_function() {
return '<div id="myDiv">Click me!</div>';
}
add_shortcode('my_shortcode', 'my_shortcode_function');
In this example, the shortcode will output a div with the ID “myDiv”. When this div is clicked, it will trigger the JavaScript function defined in “custom.js”.
Make the Custom Shortcode File Accessible
To ensure WordPress recognizes your new shortcode, include the “custom-shortcode.php” file in your theme’s functions.php file:
include get_template_directory() . '/custom-shortcode.php';
Add the Shortcode to WordPress Pages or Posts
To add a shortcode to a WordPress page or post, edit the post or page where you want to add the shortcode in the WordPress dashboard. Then, add a new “Shortcode” Gutenberg block. You can then type your shortcode into the block:
[my_shortcode]
Enqueue the JavaScript File for your Shortcode
Finally, enqueue your JavaScript file using the wp_enqueue_script
function in your “custom-shortcode.php” file:
function my_enqueue_scripts() {
wp_enqueue_script('my-script', get_template_directory_uri() . '/custom.js', array('jquery'), '1.0.0', true);
}
add_action('wp_enqueue_scripts', 'my_enqueue_scripts');
This tells WordPress to load your JavaScript file when it loads your shortcode. Now, your shortcode is not only outputting HTML, but also interacting with JavaScript to create a dynamic user experience!
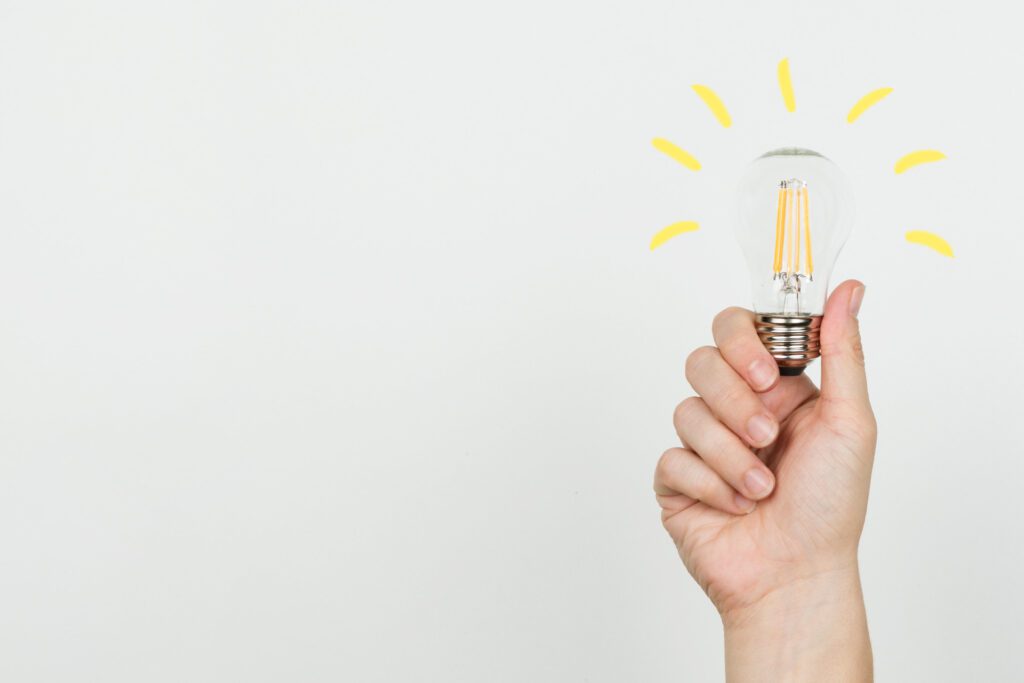
JavaScript For WordPress Tips and Tricks
When enhancing WordPress shortcodes with JavaScript, it’s important to follow best practices to ensure your code is efficient, maintainable, and compatible with other WordPress plugins and themes. Now that you understand the basics, here are some WordPress JavaScript tips and common mistakes to avoid:
Tips For Using JavaScript to Create WordPress Shortcodes
Enqueue your scripts: Always use WordPress’s built-in wp_enqueue_script
function to add your JavaScript files to your theme or plugin. This ensures that your scripts are loaded in the correct order and prevents conflicts with other plugins and themes.
Use AJAX for dynamic content: If your shortcode needs to fetch dynamic content, consider using AJAX. This allows your shortcode to retrieve and display new data without requiring the user to refresh the page.
Keep your JavaScript and PHP separate: While it’s possible to output JavaScript directly from your PHP shortcode function, it’s generally better to keep your JavaScript in separate .js files. This makes your code easier to manage and debug.
Common Mistakes to Avoid When Using JavaScript over PHP
Don’t forget to include your shortcode file: If you’re defining your shortcode in a separate PHP file, don’t forget to include this file in your theme’s functions.php file. This ensures that WordPress knows about your shortcode and can execute it when needed.
Avoid direct database queries: When fetching data for your shortcode, avoid making direct database queries. Instead, use WordPress’s built-in functions and APIs. This ensures your code is secure and compatible with all WordPress installations.
Don’t rely on shortcodes for layout: While it can be tempting to use shortcodes to create complex layouts, this can make your content difficult to manage and can lead to compatibility issues with different themes. Instead, consider using a page builder plugin, a theme with built-in layout options, or custom Gutenberg blocks.
Best Practices for Optimizing the Performance of Your Shortcodes
Only load scripts when needed: To improve performance, only load your JavaScript files on the pages that actually use your shortcode. You can do this by checking if your shortcode is used in a post before enqueuing your scripts.
Minimize your JavaScript: To reduce load times, consider minifying your JavaScript files. This removes unnecessary whitespace and comments, making your files smaller and faster to download.
Use caching: If your shortcode fetches dynamic content, consider using a caching plugin to store and reuse this data. This can significantly improve the performance of your shortcode.
Final Thoughts
Throughout this article, we’ve journeyed through the process of enhancing WordPress shortcodes with JavaScript. We’ve learned how to create a JavaScript file and how to create a shortcode in WordPress. We’ve also discovered how to make the custom shortcode file accessible, how to add the shortcode to WordPress pages or posts, and the importance of enqueueing JavaScript for a shortcode.
We’ve compared JavaScript and PHP in the context of WordPress shortcodes, highlighting the advantages of using JavaScript over PHP. These advantages include speed and performance, flexibility and control, interactivity and user experience, and compatibility with modern web technologies.
Moreover, we’ve shared some best practices and tips for using JavaScript to enhance WordPress shortcodes, as well as common mistakes to avoid. These insights will help you optimize the performance of your shortcodes and ensure your code is efficient, maintainable, and compatible with other WordPress plugins and themes.
Now, it’s your turn to experiment with enhancing WordPress shortcodes with JavaScript. Don’t be afraid to try new things and push the boundaries of what you can create. Remember, the best way to learn is by doing, and with the power of JavaScript at your disposal, there’s no limit to what you can achieve.
Using JavaScript to enhance WordPress shortcodes opens up a world of possibilities for creating dynamic, interactive content on your WordPress site. So why not give it a try? You might be surprised at what you can accomplish.
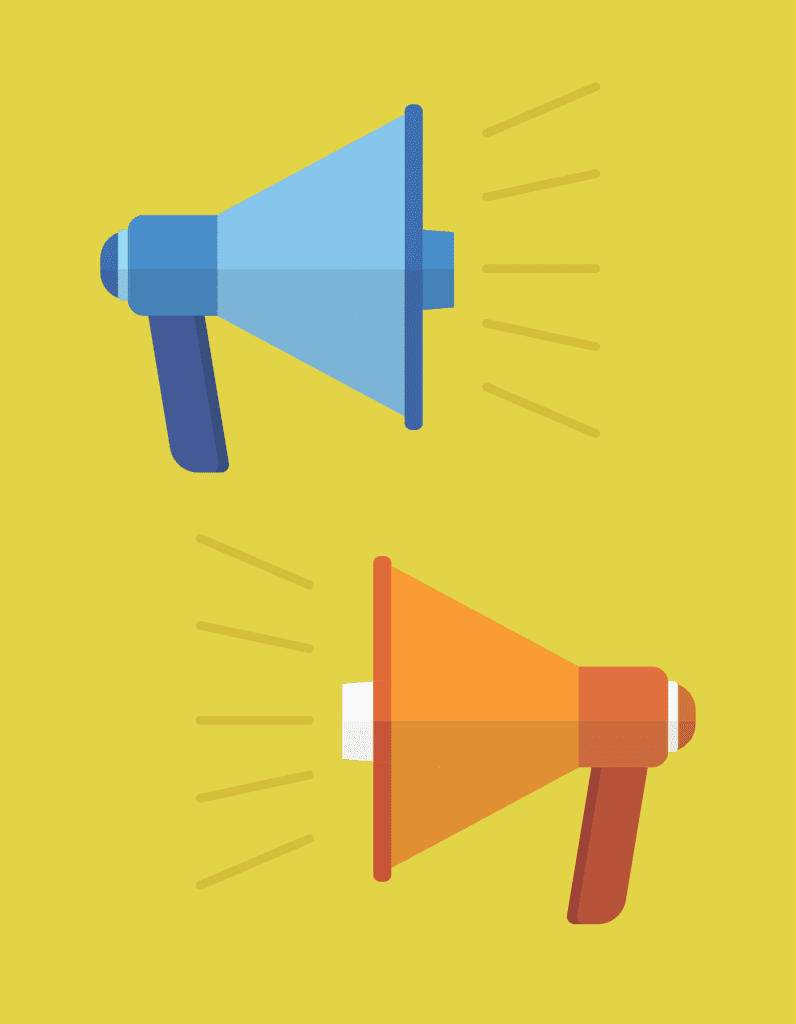
Free eBook: Headless WordPress – A New Era of Web Development
You’ve just learned how to enhance your WordPress shortcodes with the power of JavaScript. But why stop there? There’s so much more to discover and learn!
By subscribing to WordPress Whispers, you’ll stay ahead of the curve with our in-depth tools and tips. We’re all about bridging the gap between modern JavaScript and WordPress, and we want to help you supercharge your WordPress functionality.
And here’s the best part: for a limited time only, we’re offering our exclusive 28-page eBook, “Headless WordPress: A New Era of Web Development”, a $49.99 value, absolutely free upon sign-up!
Don’t miss out on this opportunity to elevate your WordPress skills. Subscribe to WordPress Whispers today!